线程池实现异步任务执行java代码实现 请给出三个样例
时间: 2023-08-31 11:04:53 浏览: 51
1. 使用Java自带的Executor框架实现线程池异步任务执行:
```
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.submit(() -> {
//执行异步任务
});
}
executor.shutdown();
```
2. 使用Spring框架的ThreadPoolTaskExecutor实现线程池异步任务执行:
```
@Autowired
private ThreadPoolTaskExecutor executor;
public void doAsyncTask() {
for (int i = 0; i < 100; i++) {
executor.execute(() -> {
//执行异步任务
});
}
}
```
3. 使用自定义的线程池实现异步任务执行:
```
public class CustomThreadPool {
private static final int THREAD_POOL_SIZE = 10;
private ExecutorService executor;
public CustomThreadPool() {
executor = new ThreadPoolExecutor(THREAD_POOL_SIZE, THREAD_POOL_SIZE,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
public void execute(Runnable task) {
executor.execute(task);
}
public void shutdown() {
executor.shutdown();
}
}
public class AsyncTaskExample {
public static void main(String[] args) {
CustomThreadPool threadPool = new CustomThreadPool();
for (int i = 0; i < 100; i++) {
threadPool.execute(() -> {
//执行异步任务
});
}
threadPool.shutdown();
}
}
```
相关推荐
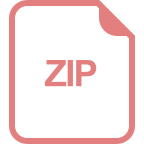














