Python改写A*算法
时间: 2023-12-04 13:51:43 浏览: 102
好的,下面是Python语言中的A*算法实现:
```
def astar(start, goal, graph):
open_list = [start]
closed_list = []
g_scores = {start: 0}
f_scores = {start: heuristic(start, goal)}
path = {}
while open_list:
current = min(f_scores, key=f_scores.get)
if current == goal:
return reconstruct_path(path, goal)
open_list.remove(current)
closed_list.append(current)
for neighbor in graph[current]:
if neighbor in closed_list:
continue
tentative_g_score = g_scores[current] + graph[current][neighbor]
if neighbor not in open_list:
open_list.append(neighbor)
elif tentative_g_score >= g_scores[neighbor]:
continue
path[neighbor] = current
g_scores[neighbor] = tentative_g_score
f_scores[neighbor] = tentative_g_score + heuristic(neighbor, goal)
return None
def reconstruct_path(path, current_node):
if current_node in path:
return reconstruct_path(path, path[current_node]) + [current_node]
else:
return [current_node]
def heuristic(node, goal):
return abs(node[0] - goal[0]) + abs(node[1] - goal[1])
```
在这个实现中,我们使用了一个open_list和一个closed_list来跟踪已经访问过的节点和待访问的节点。我们还使用了g_scores和f_scores字典来跟踪每个节点的已知最短距离和估计距离。最后,我们使用一个path字典来跟踪到达每个节点的最短路径。
在函数astar中,我们首先将起始节点添加到open_list中,并将其g_score设置为0,f_score设置为启发式估计值。然后我们进入一个while循环,直到open_list为空或者我们找到了目标节点。在循环中,我们选择open_list中f_score最小的节点,并将其从open_list中删除并添加到closed_list中。然后我们遍历当前节点的所有邻居,并计算到达邻居节点的距离。如果邻居节点已经在closed_list中,我们就跳过这个邻居节点;否则,我们将其添加到open_list中,并更新路径和距离信息。最后,如果我们没有找到目标节点,就返回None。
在函数reconstruct_path中,我们使用path字典递归地重建到达目标节点的最短路径。
在函数heuristic中,我们使用曼哈顿距离作为启发式估计函数。
阅读全文
相关推荐
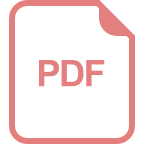
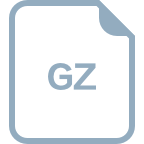
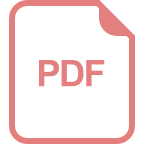
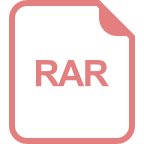
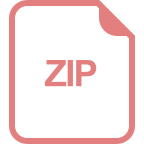
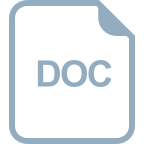
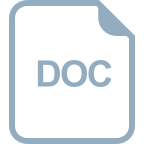
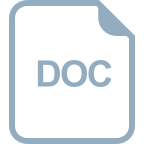
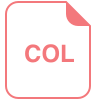






