用C# 写一个socketudp接收超时重发数据的方法
时间: 2023-08-14 11:03:45 浏览: 140
以下是一个C#的UDP接收超时重发方法的示例代码:
```csharp
public static void ReceiveWithRetry(UdpClient client, int timeout, int maxAttempts)
{
int attempts = 0;
bool received = false;
IPEndPoint endpoint = null;
byte[] buffer = new byte[1024];
while (!received && attempts < maxAttempts)
{
try
{
client.Client.ReceiveTimeout = timeout;
// 接收数据
buffer = client.Receive(ref endpoint);
Console.WriteLine("Received data from {0}", endpoint.ToString());
// 标记已经接收到数据
received = true;
}
catch (SocketException ex)
{
if (ex.SocketErrorCode == SocketError.TimedOut)
{
// 超时重试
attempts++;
Console.WriteLine("Receive timed out, retrying ({0}/{1})", attempts, maxAttempts);
}
else
{
// 其他错误抛出异常
throw ex;
}
}
}
if (!received)
{
// 接收失败,抛出异常或进行其他处理
Console.WriteLine("Failed to receive data after {0} attempts", maxAttempts);
}
}
```
该方法接受三个参数:
- `client`:`UdpClient`对象,用于接收数据。
- `timeout`:超时时间,以毫秒为单位。
- `maxAttempts`:最大重试次数。
该方法会在超时时进行重试,最多重试 `maxAttempts` 次。如果在所有尝试中都没有接收到数据,则会抛出异常或进行其他处理。
注意:该方法只能在接收数据时进行重试,无法在发送数据时进行重试。如果需要在发送数据时进行重试,需要自行编写重试逻辑。
阅读全文
相关推荐



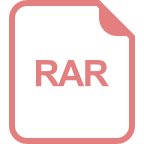
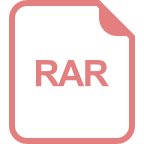
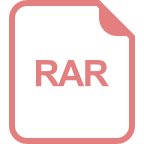
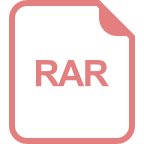
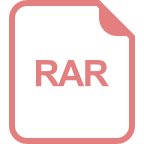
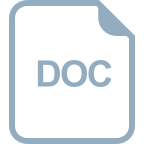
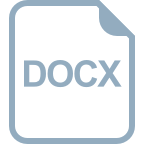
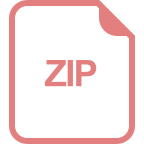