jt/t808V2011消息结构Java实现
时间: 2023-07-11 07:49:30 浏览: 49
以下是一个简单的JT/T808-2011消息结构的Java实现示例,以位置信息汇报消息为例:
```java
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.Arrays;
// 定义消息头格式
class MessageHeader {
private int msgId; // 消息ID
private int msgAttr; // 消息体属性
private String phoneNum; // 终端手机号
private int msgSeq; // 消息流水号
public MessageHeader(int msgId, int msgAttr, String phoneNum, int msgSeq) {
this.msgId = msgId;
this.msgAttr = msgAttr;
this.phoneNum = phoneNum;
this.msgSeq = msgSeq;
}
public byte[] toBytes() {
ByteBuffer buffer = ByteBuffer.allocate(12).order(ByteOrder.BIG_ENDIAN);
buffer.putShort((short) msgId);
buffer.putShort((short) msgAttr);
buffer.put(phoneNum.getBytes());
buffer.putShort((short) msgSeq);
return buffer.array();
}
}
// 定义位置信息汇报消息体格式
class LocationReport {
private byte warningFlag; // 报警标识
private int statusFlag; // 状态标识
private int lat; // 纬度,单位为1/10^6度
private int lon; // 经度,单位为1/10^6度
private short altitude; // 海拔高度,单位为米
private short speed; // 速度,单位为千米/小时
private short direction; // 方向,单位为度
private int timestamp; // 时间戳
public LocationReport(byte warningFlag, int statusFlag, int lat, int lon,
short altitude, short speed, short direction, int timestamp) {
this.warningFlag = warningFlag;
this.statusFlag = statusFlag;
this.lat = lat;
this.lon = lon;
this.altitude = altitude;
this.speed = speed;
this.direction = direction;
this.timestamp = timestamp;
}
public byte[] toBytes() {
ByteBuffer buffer = ByteBuffer.allocate(28).order(ByteOrder.BIG_ENDIAN);
buffer.put(warningFlag);
buffer.putInt(statusFlag);
buffer.putInt(lat);
buffer.putInt(lon);
buffer.putShort(altitude);
buffer.putShort(speed);
buffer.putShort(direction);
buffer.putInt(timestamp);
return buffer.array();
}
}
// 构造位置信息汇报消息
int msgId = 0x0200; // 消息ID
int msgAttr = 0x0000; // 消息体属性
String phoneNum = "123456789012"; // 终端手机号
int msgSeq = 1; // 消息流水号
MessageHeader header = new MessageHeader(msgId, msgAttr, phoneNum, msgSeq); // 消息头
byte warningFlag = 0x00; // 报警标识
int statusFlag = 0x0001; // 状态标识
int lat = 116300000; // 纬度,单位为1/10^6度
int lon = 40000000; // 经度,单位为1/10^6度
short altitude = 1000; // 海拔高度,单位为米
short speed = 60; // 速度,单位为千米/小时
short direction = 90; // 方向,单位为度
int timestamp = 1234567890; // 时间戳
LocationReport location = new LocationReport(warningFlag, statusFlag, lat, lon,
altitude, speed, direction, timestamp); // 消息体
byte[] msgBody = location.toBytes(); // 将消息体打包为字节数组
int msgLength = msgBody.length; // 消息体长度
msgAttr |= msgLength; // 更新消息体属性中的消息体长度
header = new MessageHeader(msgId, msgAttr, phoneNum, msgSeq); // 更新消息头
byte[] msg = ByteBuffer.allocate(12 + msgLength + 2).order(ByteOrder.BIG_ENDIAN)
.put(header.toBytes()).put(msgBody).array(); // 将消息头和消息体组合为完整消息
byte checksum = 0;
for (byte b : msg) {
checksum ^= b; // 计算校验码
}
msg = ByteBuffer.allocate(msg.length + 2).order(ByteOrder.BIG_ENDIAN)
.put(msg).put(checksum).put((byte) 0x7e).array(); // 将校验码和消息尾部添加到消息中
// 发送消息
sendMsg(msg);
```
以上代码仅为示例,实际应用中需要根据具体需求进行修改和完善。
相关推荐
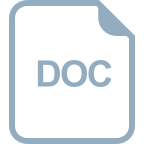
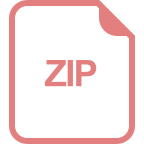













