帮我写一个C++代码相似性检测的程序
时间: 2023-03-29 08:04:29 浏览: 150
你好,C 代码相似性检测可以使用字符串匹配和算法来实现。以下是一个简单的示例程序,可以比较两个C代码文件之间的相似性。
```c
#include <stdio.h>
#include <string.h>
#define MAXSIZE 10000
void remove_comments(char *str)
{
int inside_comment = 0;
char *p = str;
char *q = str;
while (*p != '\0') {
if (!inside_comment) {
if (*p == '/' && *(p+1) == '*') {
inside_comment = 1;
p++;
} else if (*p == '/' && *(p+1) == '/') {
*q++ = '\n';
*q++ = '\0';
break;
} else {
*q++ = *p;
}
} else if (*p == '*' && *(p+1) == '/') {
inside_comment = 0;
p++;
}
p++;
}
*q = '\0';
}
int main()
{
char code1[MAXSIZE];
char code2[MAXSIZE];
char line1[MAXSIZE];
char line2[MAXSIZE];
printf("Enter the first code:\n");
fgets(code1, MAXSIZE, stdin);
printf("Enter the second code:\n");
fgets(code2, MAXSIZE, stdin);
// Remove comments from both codes
remove_comments(code1);
remove_comments(code2);
int lines1 = 0, lines2 = 0, similar = 0;
// Split the code into lines and compare each line
char *token1 = strtok(code1, "\n");
while (token1 != NULL) {
lines1++;
char *token2 = strtok(code2, "\n");
while (token2 != NULL) {
lines2++;
if (strcmp(token1, token2) == 0) {
similar++;
}
token2 = strtok(NULL, "\n");
}
token1 = strtok(NULL, "\n");
}
printf("Similarity: %d/%d (%.2f%%)\n", similar, lines1, (float)similar/lines1*100);
return 0;
}
```
这个程序会提示用户输入两个C代码文件,并将其存储在两个字符数组中。然后,它会使用`remove_comments()`函数删除每个代码中的注释。接下来,它将每个代码分割成单独的行,并将它们逐行进行比较,检测它们之间的相似性。
请注意,这个程序只是一个简单的示例程序,仅使用字符串匹配算法来比较代码的相似性。更复杂的算法和技术,如词频分析、语法分析和机器学习等,可以用来进一步提高相似性检测的准确性。
阅读全文
相关推荐
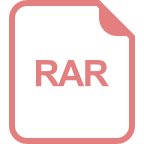

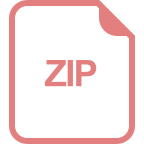
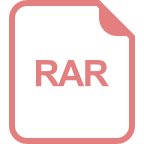
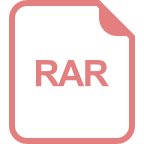
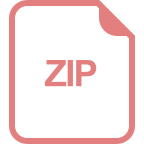
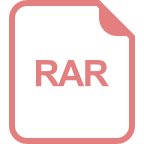
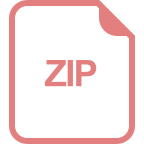
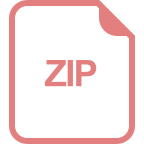
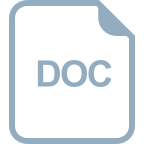
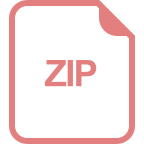
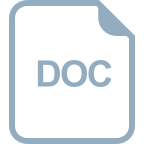
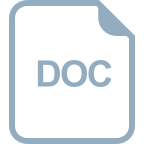
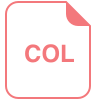
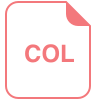