if not os.path.exists(dst_path): os.makedirs(dst_path)
时间: 2024-09-18 21:12:21 浏览: 91
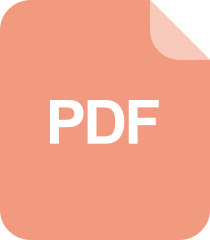
Python对文件和目录进行操作的方法(file对象/os/os.path/shutil 模块)
`os.makedirs()`函数用于创建目录树,如果指定的路径不存在,则会递归地创建所需的各级目录。这个函数在处理文件复制或移动时非常有用,特别是在需要保证目标路径存在的情况下。
如果你想要演示`if not os.path.exists(dst_path): os.makedirs(dst_path)`这一条件语句的作用,可以按照下面的方式操作:
```python
# 假设我们有一个源文件夹src_path,你想将其中的内容复制到dst_path,但只有当dst_path不存在时才创建它
import shutil
src_path = "source_directory"
dst_path = "destination_directory"
if not os.path.exists(dst_path): # 检查dst_path是否存在
os.makedirs(dst_path) # 如果不存在则创建目录
# 然后你可以使用shutil.copytree()或shutil.move()来复制或移动文件
shutil.copytree(src_path, dst_path)
# 或者使用shutil.rmtree()来删除源目录(仅示例,实际应用需谨慎)
# shutil.rmtree(src_path)
```
这段代码会在`dst_path`不存在时自动创建它,然后再进行后续的操作。
阅读全文
相关推荐
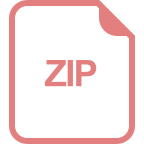
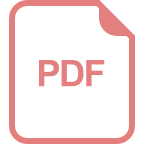











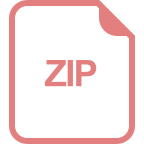
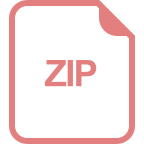
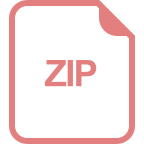
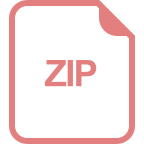