模拟退火算法求解01背包问题python
时间: 2023-09-06 16:13:47 浏览: 224
好的,我可以为您提供一个基于 Python 的模拟退火算法求解 01 背包问题的实现。
首先,我们需要定义背包问题中的一些参数,包括物品数量、背包容量、每个物品的重量和价值等。假设这些参数分别为 `num_items`、`capacity`、`weights` 和 `values`。
接下来,我们可以定义一个函数来计算当前方案的总价值:
```python
def calc_value(solution, values):
return sum(solution[i] * values[i] for i in range(len(solution)))
```
然后,我们可以定义一个函数来生成一个随机的初始解:
```python
import random
def generate_random_solution(num_items):
return [random.randint(0, 1) for _ in range(num_items)]
```
接着,我们可以定义一个函数来执行模拟退火算法:
```python
import math
def simulated_annealing(num_items, capacity, weights, values, initial_temperature=100, cooling_rate=0.99, stopping_temperature=0.1):
# 初始化当前最优解
best_solution = None
best_value = 0
# 初始化当前解
current_solution = generate_random_solution(num_items)
current_value = calc_value(current_solution, values)
# 初始化温度
temperature = initial_temperature
# 开始迭代
while temperature > stopping_temperature:
# 生成随机解
next_solution = generate_random_solution(num_items)
next_value = calc_value(next_solution, values)
# 计算能量差
energy_diff = next_value - current_value
# 判断是否接受更差的解
if energy_diff > 0:
# 更好的解,直接接受
current_solution = next_solution
current_value = next_value
# 更新最优解
if current_value > best_value:
best_solution = current_solution
best_value = current_value
else:
# 更差的解,以一定概率接受
acceptance_prob = math.exp(energy_diff / temperature)
if random.random() < acceptance_prob:
current_solution = next_solution
current_value = next_value
# 降低温度
temperature *= cooling_rate
return best_solution, best_value
```
最后,我们可以使用上述函数来解决 01 背包问题:
```python
num_items = 10
capacity = 50
weights = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
values = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
best_solution, best_value = simulated_annealing(num_items, capacity, weights, values)
print("Best solution:", best_solution)
print("Best value:", best_value)
```
这样,我们就可以得到一个随机的最优解,它代表了一个能够放入背包中的物品组合,使得总价值最大。
阅读全文
相关推荐
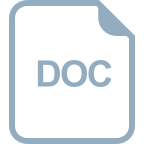
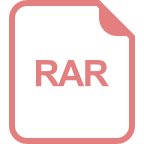
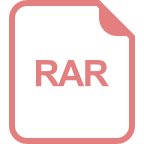









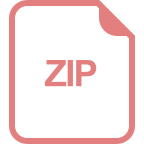
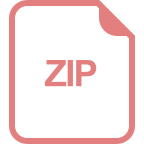
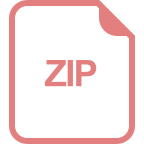
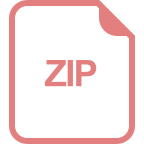
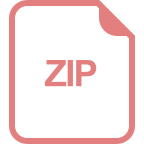
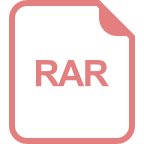
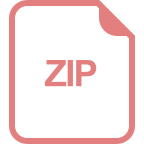
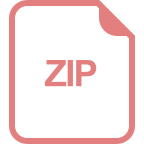