C++GUI电子时钟
时间: 2024-08-17 20:02:56 浏览: 53
C++ GUI电子时钟是一种使用图形用户界面(GUI)库在C++编程语言中创建的电子时钟应用程序。在C++中实现GUI应用程序,通常需要使用第三方库,比如Qt、wxWidgets或FLTK。这些库提供了丰富的控件和接口,使得开发者能够设计和实现用户界面。
以Qt为例,它是一个跨平台的C++框架,用于开发GUI应用程序。一个简单的电子时钟可能包含一个显示时间的标签(QLabel)以及一个定时器(QTimer)用于更新时间。以下是一个基本的C++ GUI电子时钟的示例步骤:
1. 初始化GUI环境:创建一个Qt应用程序实例。
2. 创建窗口:定义一个继承自QWidget的类,并在其中设置布局和控件。
3. 创建显示时间的标签:添加一个QLabel到窗口中用于显示时间。
4. 实现定时器:使用QTimer设置一个时间间隔(例如1000毫秒),并在定时器的timeout信号触发时更新标签内容。
5. 连接信号和槽:将定时器的timeout信号连接到一个槽函数,该槽函数会获取当前时间并更新标签的文本。
6. 显示窗口:执行窗口的显示方法,启动事件循环。
下面是一个简单的代码框架,展示了如何使用Qt创建一个电子时钟:
```cpp
#include <QApplication>
#include <QWidget>
#include <QLabel>
#include <QTimer>
#include <QVBoxLayout>
#include <QDateTime>
class ClockWidget : public QWidget {
public:
ClockWidget(QWidget *parent = nullptr) : QWidget(parent) {
// 设置布局
QVBoxLayout *layout = new QVBoxLayout(this);
// 创建时间显示标签
timeLabel = new QLabel("00:00:00", this);
layout->addWidget(timeLabel);
// 创建定时器
QTimer *timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &ClockWidget::updateTime);
// 启动定时器
timer->start(1000);
}
protected:
void updateTime() {
// 获取当前时间并更新标签
timeLabel->setText(QDateTime::currentDateTime().toString("HH:mm:ss"));
}
private:
QLabel *timeLabel;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
ClockWidget clockWidget;
clockWidget.show();
return app.exec();
}
```
阅读全文
相关推荐
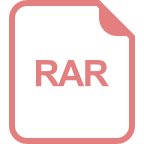
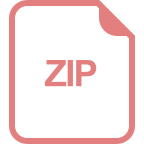
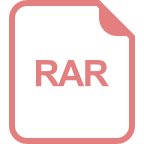
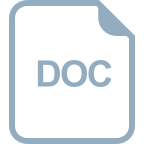
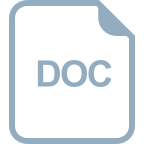
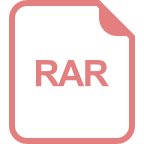
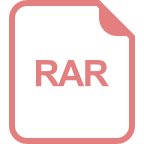
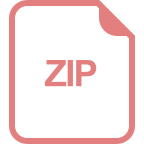
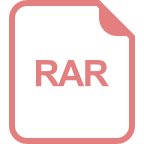
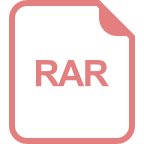
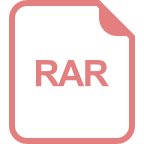
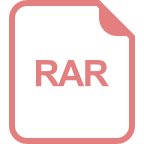
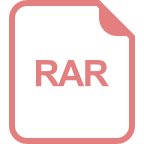
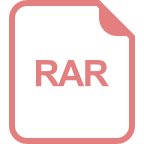
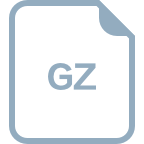
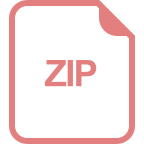
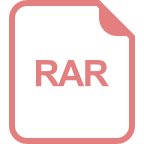
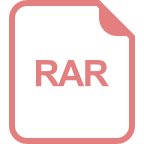