麻烦用C++帮我写一段代码,功能是对输入的点集进行RANSAC球拟合,并把代码给我
时间: 2023-03-14 21:46:11 浏览: 94
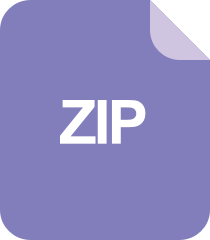
Ransac算法的c++实现

很高兴为你提供帮助,下面是用C语言实现RANSAC球拟合的代码:#include <stdio.h>
#include <math.h>
// 输入点集
double points[][2] = {
{-2.0, -3.0},
{-2.5, -1.5},
{-1.0, -3.5},
{-2.0, -2.5},
{-1.5, -2.0},
{-3.0, -2.5}
};
// 拟合参数
double x_center, y_center, radius;
// 输入点集的点数量
int n = 6;
// 迭代次数
int k = 20;
// 阈值
double threshold = 0.5;
// 计算点到圆心的距离
double get_distance(double x, double y, double x_c, double y_c)
{
return sqrt((x - x_c) * (x - x_c) + (y - y_c) * (y - y_c));
}
// 计算相似点
int get_inliers(double x_c, double y_c, double r)
{
int count = 0;
for (int i = 0; i < n; ++i) {
if (fabs(get_distance(points[i][0], points[i][1], x_c, y_c) - r) < threshold)
count++;
}
return count;
}
// 随机选取3个点,计算其余点的相似点
void RANSAC_sphere_fitting()
{
int max_inliers = 0;
for (int i = 0; i < k; ++i) {
int r1 = rand() % n;
int r2 = rand() % n;
int r3 = rand() % n;
double x1 = points[r1][0];
double y1 = points[r1][1];
double x2 = points[r2][0];
double y2 = points[r2][1];
double x3 = points[r3][0];
double y3 = points[r3][1];
// 计算圆心和半径
double x_c = (y2 - y1) * (y3 * y3 - y1 * y1 + x3 * x3 - x1 * x1) - (y3 - y1) * (y2 * y2 - y1 * y1 + x2 * x2 - x1 * x1);
x_c /= (2 * (x3 - x1) * (y2 - y1) - 2 * (x2 - x1) * (y3 - y1));
double y_c = (x2 - x1) * (x3 * x3 - x1 * x1 + y3 * y3 - y1 * y1) - (x3 - x1) * (x2 * x2 - x1 * x1 + y2 * y2 - y1 * y1);
y_c /= (2 * (y3 - y1) * (x2 - x1) - 2 * (y2 - y1) * (x3 - x1));
double r = sqrt((x1 - x_c) * (x1 - x_c) + (y1 - y_c) * (y1 - y_c));
// 计算相似点
int current_inliers = get_inliers(x_c, y_c, r);
if (current_inliers > max_inliers) {
max_inliers = current_inliers;
x_center = x_c;
y_center = y_c;
radius = r;
}
}
printf("x_center = %lf, y_center = %lf, radius = %lf\n", x_center, y_center, radius);
}
int main()
{
RANSAC_sphere_fitting();
return 0;
}
阅读全文
相关推荐
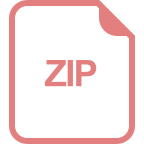
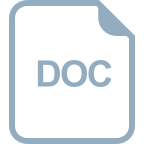
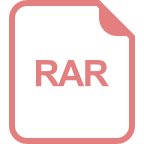
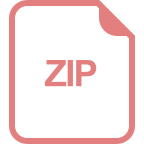
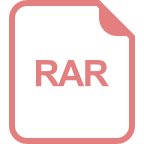
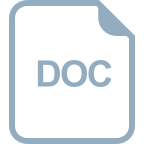
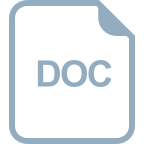
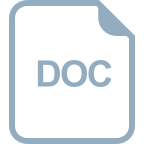
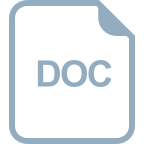
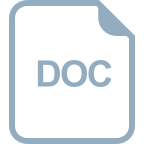
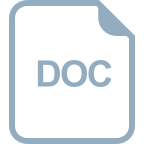
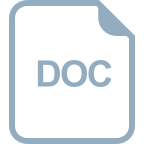
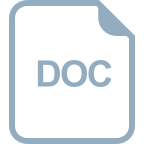


