怎样在@RequestMapping注解上找到接口的路径
时间: 2023-08-03 07:57:04 浏览: 108
要找到@RequestMapping注解上的接口路径,你可以按照以下步骤进行操作:
1. 打开你的Spring Boot项目。
2. 找到包含你要调试的接口的控制器类(通常是带有@Controller或@RestController注解的类)。
3. 在控制器类中找到你想要调试的具体方法,该方法应该带有@RequestMapping注解。
4. 查看@RequestMapping注解上的value属性或path属性。这些属性指定了接口的路径。
例如,如果你的控制器类如下所示:
```java
@RestController
@RequestMapping("/api")
public class UserController {
@PostMapping("/user")
public ResponseEntity<User> createUser(@RequestBody User user) {
// 处理创建用户的逻辑
}
}
```
在这个例子中,@RequestMapping注解上的路径是`/api`,而@PostMapping注解上的路径是`/user`。因此,完整的接口路径是`/api/user`。
通过这种方式,你可以在控制器类中找到@RequestMapping注解上的接口路径。
相关问题
如何确认是否有正确的@RequestMapping注解和对应的Feign接口方法,该方法期望接收或返回指定的返回类型。
确认有正确的`@RequestMapping`注解和对应Feign接口方法的主要步骤如下:
1. **检查接口**: 打开你正在使用的Feign接口文件,查看是否存在一个或者多个公开的(通常使用`@GetMapping`、`@PostMapping`、`@PutMapping`、`@DeleteMapping`等注解)方法。这些方法通常会映射到API端点上,并且有明确的请求方法(GET、POST等)、路径以及可能的参数。
```java
@GetMapping("/api/v1/users")
public ReturnJSON<Boolean> getUsers();
```
在上面的例子中,`@GetMapping`表明这是一个HTTP GET请求,`/api/v1/users`是请求的路径,返回类型是`ReturnJSON<Boolean>`。
2. **检查返回类型**:查看方法声明,确保其返回值类型是你期待的类型。比如,如果返回类型是`ReturnJSON<Boolean>`,那么方法应声明为返回这种类型的实例。
3. **检查注解参数**: `@RequestMapping`注解可能有一些额外的属性,如`params`, `headers`, 或 `consumes` 和 `produces` 来指定特定的请求参数和媒体类型。确认这些属性是否匹配实际的API需求。
4. **验证实际API文档**:参考你正在集成的后端API文档,确保`@RequestMapping`的方法、URL、HTTP方法及返回类型都一致。
如果发现不匹配的地方,你需要更新Feign接口或者调整后端API的设计以匹配。
服务端@PostMapping接口未接收到feign接口通过@RequestMapping修饰的接口信息
这个问题可能有多种原因导致,以下是一些可能的原因和解决方法:
1. 检查服务端的@PostMapping接口是否与feign接口通过@RequestMapping修饰的接口的请求参数一致,包括请求方法、请求路径、请求头、请求体等信息。
2. 检查服务端的@PostMapping接口是否正确地接收了请求体中的参数。如果请求体中的参数是JSON格式,需要使用@RequestBody注解将其转换成Java对象。
3. 检查服务端的@PostMapping接口是否正确地解析了feign接口通过@RequestMapping修饰的接口的响应结果。如果响应结果是JSON格式,需要使用@ResponseBody注解将其转换成JSON格式。
4. 检查服务端的@PostMapping接口是否存在异常处理机制。如果feign接口通过@RequestMapping修饰的接口返回的响应码不是200,服务端应该对异常进行处理,例如返回自定义的错误信息。
5. 检查服务端和feign接口通过@RequestMapping修饰的接口之间的网络连接是否正常。可以使用网络抓包工具检查请求和响应的数据包是否正常发送和接收。
阅读全文
相关推荐
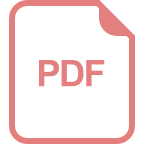
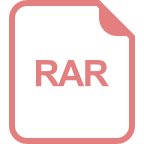

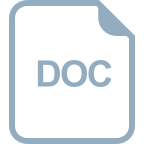
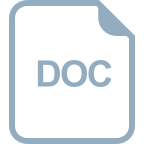










