使用uniapp并使用vue3语法做一个圆形进度条进行交互
时间: 2024-09-09 18:00:47 浏览: 67
使用 uni-app 开发一个圆形进度条,并结合 Vue3 的语法,你可以按照以下步骤操作:
1. 首先,在项目中引入 Vue 和相关的组件库,如 `vant` 或者自定义的 CSS 进度条组件。你可以使用 npm 安装 vant 组件:
```bash
npm install vant --save
```
2. 创建一个 Vue 文件,比如 `CircularProgress.vue`,并将 Vue3 的语法结构添加到其中:
```html
<template>
<view class="progress-circle">
<van-progress :percentage="progressPercentage" :stroke-width="strokeWidth" type="circle"></van-progress>
</view>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const progressPercentage = ref(0); // 进度百分比,可以通过绑定值来控制进度
const strokeWidth = ref(5); // 圈形边框宽度
return {
progressPercentage,
strokeWidth,
};
},
};
</script>
<style scoped>
.progress-circle {
width: 100px;
height: 100px;
border-radius: 50%;
position: relative;
}
</style>
```
3. 在父组件或需要使用圆形进度条的地方导入并使用这个组件,通过 `:value` 或者 `v-model` 绑定数据改变进度:
```html
<template>
<view>
<CircularProgress v-bind:progressPercentage="currentProgress" />
</view>
</template>
<script>
import CircularProgress from '@/components/CircularProgress.vue';
export default {
components: {
CircularProgress,
},
data() {
return {
currentProgress: 50, // 初始进度为50%
};
},
};
</script>
```
4. 对于交互部分,你可以监听 `input` 事件来自定义进度变化或用户点击事件更新进度。例如:
```javascript
setup() {
...
watch('currentProgress', (newValue) => {
progressPercentage.value = newValue; // 更新组件显示的新进度
});
onMounted(() => {
// 可能的点击事件处理
this.$el.addEventListener('click', () => {
this.currentProgress += 10; // 点击增加10%进度
});
});
...
}
```
阅读全文
相关推荐
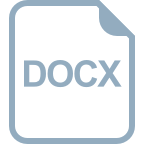
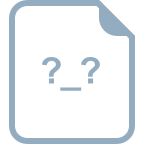
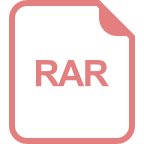
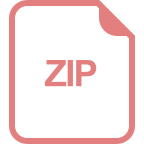
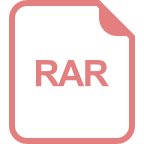
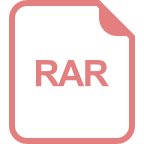
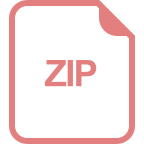
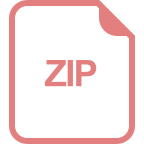
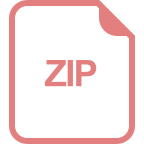
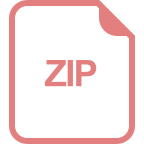
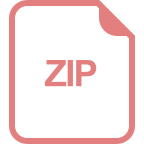
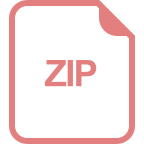
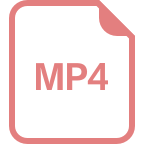
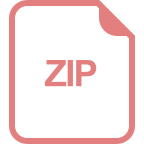
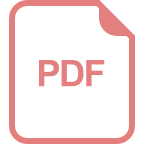
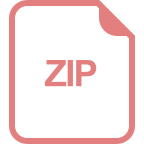
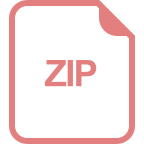