基于opencv的简单p图软件,具备一些常用的P图功能例如彩色图像转黑白图像,明暗亮度变化,反色处理,磨皮,生成素描等,主要包含三个部分:上传图片部分,P图部分,保存结果部分。
时间: 2023-12-07 10:03:07 浏览: 38
以下是一个基于OpenCV的简单P图软件,具备一些常用的P图功能,包含上传图片部分,P图部分和保存结果部分:
```python
import cv2
import numpy as np
import tkinter as tk
from tkinter import filedialog
# 创建GUI窗口
root = tk.Tk()
root.geometry("400x400")
# 定义函数:上传图片
def select_image():
global img, img_original
file_path = filedialog.askopenfilename()
img_original = cv2.imread(file_path)
img = img_original.copy()
cv2.imshow("Original Image", img_original)
# 定义函数:黑白化
def to_gray():
global img
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imshow("Result", img)
# 定义函数:明暗调整
def adjust_brightness(value):
global img
img = cv2.convertScaleAbs(img_original, alpha=1, beta=value)
cv2.imshow("Result", img)
# 定义函数:反色处理
def invert_color():
global img
img = cv2.bitwise_not(img)
cv2.imshow("Result", img)
# 定义函数:磨皮
def smoothing(value):
global img
img = cv2.GaussianBlur(img, (value, value), 0)
cv2.imshow("Result", img)
# 定义函数:生成素描
def sketch():
global img
img_gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
img_invert = cv2.bitwise_not(img_gray)
img_blur = cv2.GaussianBlur(img_invert, (21, 21), 0, 0)
img_blend = cv2.divide(img_gray, 255 - img_blur, scale=256)
img = cv2.cvtColor(img_blend, cv2.COLOR_GRAY2BGR)
cv2.imshow("Result", img)
# 定义函数:保存结果
def save_image():
file_path = filedialog.asksaveasfilename(defaultextension=".jpg")
cv2.imwrite(file_path, img)
# 创建界面元素:上传图片按钮
button_select_image = tk.Button(root, text="Select Image", command=select_image)
button_select_image.pack(fill="x")
# 创建界面元素:黑白化按钮
button_to_gray = tk.Button(root, text="To Gray", command=to_gray)
button_to_gray.pack(fill="x")
# 创建界面元素:明暗调整滑动条
scale_brightness = tk.Scale(root, from_=-255, to=255, orient=tk.HORIZONTAL, command=adjust_brightness)
scale_brightness.pack(fill="x")
# 创建界面元素:反色处理按钮
button_invert_color = tk.Button(root, text="Invert Color", command=invert_color)
button_invert_color.pack(fill="x")
# 创建界面元素:磨皮滑动条
scale_smoothing = tk.Scale(root, from_=1, to=15, orient=tk.HORIZONTAL, command=smoothing)
scale_smoothing.pack(fill="x")
# 创建界面元素:生成素描按钮
button_sketch = tk.Button(root, text="Sketch", command=sketch)
button_sketch.pack(fill="x")
# 创建界面元素:保存结果按钮
button_save_image = tk.Button(root, text="Save Image", command=save_image)
button_save_image.pack(fill="x")
# 显示界面
root.mainloop()
```
该软件实现了上传图片、黑白化、明暗调整、反色处理、磨皮、生成素描和保存结果等常用的P图功能。您可以根据需要添加更多的功能和界面元素。
相关推荐
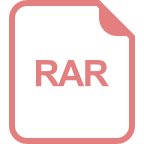
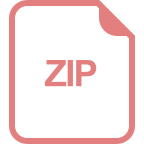














