用QT5写一个可以在电脑上通过TCP客户端将指定文件发送到服务器的工程并给出.pro .h .cpp文件
时间: 2024-02-06 11:10:38 浏览: 103
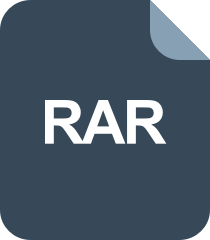
QT实现TCP通信(客户端和服务器同时包含在一个cpp文件中)
以下是一个简单的QT5工程,实现通过TCP客户端将指定文件发送到服务器:
.pro文件:
```
QT += core network
QT -= gui
TARGET = FileSender
CONFIG += console
CONFIG -= app_bundle
TEMPLATE = app
SOURCES += main.cpp \
tcpclient.cpp
HEADERS += \
tcpclient.h
```
tcpclient.h文件:
```cpp
#ifndef TCPCLIENT_H
#define TCPCLIENT_H
#include <QObject>
#include <QTcpSocket>
class TcpClient : public QObject
{
Q_OBJECT
public:
explicit TcpClient(QObject *parent = nullptr);
public slots:
void connectToServer(QString host, int port);
void sendFile(QString filePath);
private slots:
void onConnected();
void onDisconnected();
void onError(QAbstractSocket::SocketError error);
void onBytesWritten(qint64 bytes);
private:
QTcpSocket *m_socket;
};
#endif // TCPCLIENT_H
```
tcpclient.cpp文件:
```cpp
#include "tcpclient.h"
#include <QFile>
#include <QFileInfo>
#include <QDataStream>
#include <QDebug>
TcpClient::TcpClient(QObject *parent) : QObject(parent)
{
m_socket = new QTcpSocket(this);
connect(m_socket, &QTcpSocket::connected, this, &TcpClient::onConnected);
connect(m_socket, &QTcpSocket::disconnected, this, &TcpClient::onDisconnected);
connect(m_socket, &QTcpSocket::errorOccurred, this, &TcpClient::onError);
connect(m_socket, &QTcpSocket::bytesWritten, this, &TcpClient::onBytesWritten);
}
void TcpClient::connectToServer(QString host, int port)
{
m_socket->connectToHost(host, port);
}
void TcpClient::sendFile(QString filePath)
{
QFile file(filePath);
if (!file.open(QIODevice::ReadOnly))
{
qDebug() << "Failed to open file: " << file.fileName();
return;
}
QFileInfo fileInfo(file.fileName());
QString fileName(fileInfo.fileName());
qint64 fileSize = fileInfo.size();
qDebug() << "Sending file: " << fileName << " Size: " << fileSize;
QByteArray fileData;
QDataStream dataStream(&fileData, QIODevice::WriteOnly);
dataStream << fileName.toUtf8();
dataStream << fileSize;
dataStream << file.readAll();
m_socket->write(fileData);
}
void TcpClient::onConnected()
{
qDebug() << "Connected to server";
}
void TcpClient::onDisconnected()
{
qDebug() << "Disconnected from server";
}
void TcpClient::onError(QAbstractSocket::SocketError error)
{
qDebug() << "Socket error: " << error;
}
void TcpClient::onBytesWritten(qint64 bytes)
{
qDebug() << "Bytes written: " << bytes;
}
```
main.cpp文件:
```cpp
#include <QCoreApplication>
#include <QCommandLineParser>
#include "tcpclient.h"
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
QCommandLineParser parser;
parser.setApplicationDescription("File Sender - Send a file to a server via TCP.");
parser.addHelpOption();
parser.addVersionOption();
parser.addPositionalArgument("file", "The file to send.");
parser.addOption({{"h", "host"}, "The server host name.", "host"});
parser.addOption({{"p", "port"}, "The server port number.", "port", "1234"});
parser.process(app);
QString filePath = parser.positionalArguments().count() > 0 ? parser.positionalArguments().at(0) : "";
QString host = parser.isSet("host") ? parser.value("host") : "localhost";
int port = parser.isSet("port") ? parser.value("port").toInt() : 1234;
if (filePath.isEmpty())
{
qDebug() << "Please specify a file to send.";
return -1;
}
TcpClient tcpClient;
tcpClient.connectToServer(host, port);
tcpClient.sendFile(filePath);
return app.exec();
}
```
在使用之前,需要在服务器端启动一个TCP服务器。可以使用以下代码:
```cpp
QTcpServer *server = new QTcpServer(this);
connect(server, &QTcpServer::newConnection, this, &TcpServer::onNewConnection);
server->listen(QHostAddress::Any, 1234);
```
其中`onNewConnection`是处理新连接的槽函数,可以在其中接收来自客户端的文件数据并保存到本地文件中。
注意:以上代码仅作为示例,实际应用中还需要进行错误处理等相关操作。
阅读全文
相关推荐




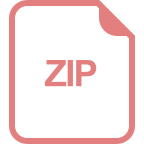
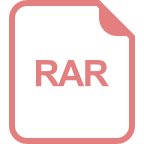
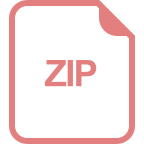
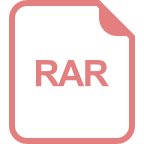
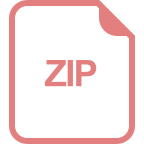
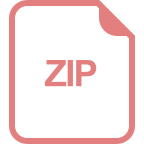
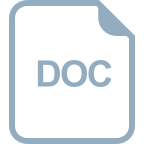
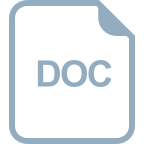
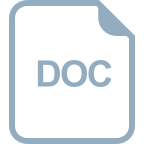
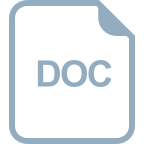
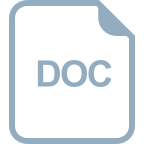
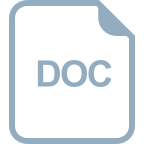