raw_list *node = new raw_list; raw_tail[idx]->pre->next = node; node->pre = raw_tail[idx]->pre; raw_tail[idx]->pre = node; node->next = raw_tail[idx]; node->isfilled = false; node->isused = false; node->data_idx = recv_data_cnt[idx]; raw_num[idx] ++; if(node->pre!=raw_head[idx]) node->pre->isfilled = true; //create new node means last one is filled
时间: 2024-03-29 08:40:53 浏览: 123
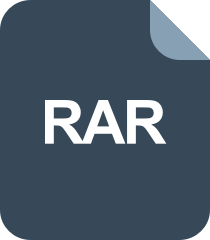
double-link-list.rar_double link list_双向链表排序
这段代码是创建一个新的节点的过程,具体实现包括以下步骤:
1. 创建一个名为node的新的raw_list类型的节点,使用new运算符动态分配内存空间。
2. 将node节点插入到raw_tail[idx]所指向的链表的倒数第二个位置(即倒数第一个节点的前一个位置)。
3. 更新node节点的前驱和后继节点的指针,以及相应的标记位和数据索引值。
4. 对于该链表中的节点数量进行统计。
5. 如果node节点的前驱节点不是链表的头节点,则将其标记为已填充(isfilled)状态。
具体代码实现可能需要结合上下文才能确认。
阅读全文
相关推荐
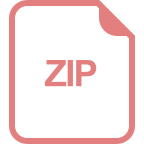
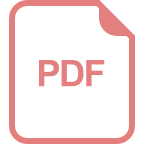












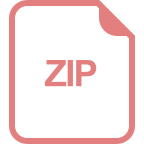
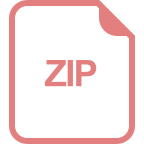