用c++实现广度优先搜索实现迷宫
时间: 2024-03-20 12:42:36 浏览: 13
好的,以下是用 C++ 实现广度优先搜索实现迷宫的代码示例:
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 定义迷宫的行数和列数
const int ROWS = 5;
const int COLS = 7;
// 定义迷宫矩阵
int maze[ROWS][COLS] = {
{0, 1, 0, 0, 0, 0, 0},
{0, 1, 0, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0}
};
// 定义起点和终点
pair<int, int> start = make_pair(0, 0);
pair<int, int> end = make_pair(ROWS - 1, COLS - 1);
// 定义前驱节点矩阵和访问标记矩阵
pair<int, int> parent[ROWS][COLS];
bool visited[ROWS][COLS] = {false};
// 定义方向数组,用于遍历相邻节点
int dx[4] = {0, 1, 0, -1};
int dy[4] = {1, 0, -1, 0};
// 定义搜索函数
vector<pair<int, int>> bfs(pair<int, int> start, pair<int, int> end) {
queue<pair<int, int>> q;
q.push(start);
visited[start.first][start.second] = true;
while (!q.empty()) {
auto curr = q.front(); q.pop();
if (curr == end) {
// 回溯路径
vector<pair<int, int>> path;
while (curr != start) {
path.push_back(curr);
curr = parent[curr.first][curr.second];
}
path.push_back(start);
reverse(path.begin(), path.end());
return path;
}
// 遍历相邻节点
for (int i = 0; i < 4; i++) {
int x = curr.first + dx[i];
int y = curr.second + dy[i];
if (x >= 0 && x < ROWS && y >= 0 && y < COLS && maze[x][y] == 0 && !visited[x][y]) {
visited[x][y] = true;
parent[x][y] = curr;
q.push(make_pair(x, y));
}
}
}
return vector<pair<int, int>>();
}
// 定义输出函数
void print_path(const vector<pair<int, int>>& path) {
for (auto node : path) {
cout << "(" << node.first << ", " << node.second << ") ";
}
cout << endl;
}
// 主函数
int main() {
auto path = bfs(start, end);
print_path(path);
return 0;
}
```
以上就是用 C++ 实现广度优先搜索实现迷宫的代码示例,希望能够对您有所帮助。
相关推荐
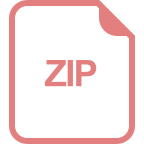
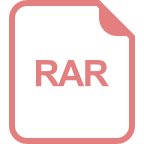
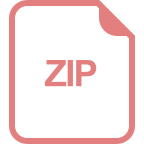














