bmp图像旋转90度c++
时间: 2023-11-03 09:16:55 浏览: 206
要将 BMP 图像逆时针旋转 90 度,可以按照以下步骤进行:
1. 读取 BMP 文件头和信息头,获取图像宽度、高度、像素位数等信息。
2. 创建一个新的 BMP 图像文件头和信息头,宽度和高度交换,颜色位数不变。
3. 计算新图像的每行像素字节数,为了保证存储空间对齐,可能需要加上填充字节。
4. 逐个读取原图像的像素行,将每个像素点按照旋转后的位置写入新图像的相应位置,注意行列互换。
5. 写入新的 BMP 文件头和信息头,保存新图像文件。
下面是一个简单的 C++ 代码实现:
```c++
#include <iostream>
#include <fstream>
#include <algorithm>
using namespace std;
#pragma pack(push, 1) // 对齐方式设为 1 字节
struct BMPHeader {
char id[2];
int size;
short reserved1, reserved2;
int offset;
int header_size;
int width, height;
short planes, bpp;
int compression, image_size;
int x_ppm, y_ppm;
int colors, important_colors;
};
#pragma pack(pop)
int main() {
const char* infile = "input.bmp";
const char* outfile = "output.bmp";
ifstream fin(infile, ios::binary);
ofstream fout(outfile, ios::binary);
BMPHeader header;
fin.read(reinterpret_cast<char*>(&header), sizeof(header));
int width = header.width;
int height = header.height;
int bpp = header.bpp;
int row_size = (width * bpp + 31) / 32 * 4; // 每行像素字节数,可能需要加上填充字节
BMPHeader new_header = header;
new_header.width = height;
new_header.height = width;
new_header.size = new_header.offset + row_size * height;
fout.write(reinterpret_cast<char*>(&new_header), sizeof(new_header));
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
char pixel[4];
fin.read(pixel, bpp / 8); // 读取一个像素
fout.seekp(new_header.offset + (height - x - 1) * row_size + y * (bpp / 8)); // 计算新位置
fout.write(pixel, bpp / 8); // 写入新像素
}
fin.ignore(row_size - width * bpp / 8); // 忽略填充字节
}
fin.close();
fout.close();
return 0;
}
```
该代码使用了结构体对齐方式设为 1 字节,确保读写文件时不会出现内存对齐问题。读取一个像素时,使用了 char 数组,其大小为像素位数的一半,因为 BMP 文件中的像素数据是按照 BGR 的顺序排列的。写入新像素时,计算新位置需要注意行列的交换。最后需要忽略原图像可能存在的填充字节。
阅读全文
相关推荐





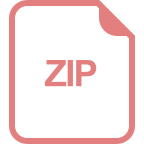





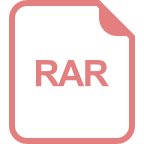


