C# EmguCV 图像模板匹配算法代码例子
时间: 2024-09-07 14:01:18 浏览: 84
Emgu CV是一个用于.NET平台的开源计算机视觉库,它提供了一个方便的方式来使用OpenCV的功能。图像模板匹配是其中一项关键技术,用于在一幅大图中寻找小图(模板)的精确位置。以下是使用Emgu CV进行模板匹配的一个基本示例:
```csharp
using Emgu.CV;
using Emgu.CV.CvEnum;
using System.Drawing;
// 假设我们有模板图片(template)
Image<Bgr, byte> template = new Image<Bgr, byte>("template.jpg");
// 要搜索的大图片(image)
Image<Bgr, byte> image = new Image<Bgr, byte>("search_image.jpg");
// 创建模板匹配器
TemplateMatchingMatch[] matches = null;
MatOfPoint2f points = new MatOfPoint2f();
RectangleF[] rectangles = new RectangleF[1];
var matcher = new TemplateMatcher();
// 使用matchTemplate方法进行匹配
matcher.Match(image, template, out matches, out points);
// 找到最佳匹配的位置
rectangles[0] = matcher.GetMinMaxLocation().ToOpenCvRect();
// 现在你可以根据需要处理这些矩形,比如打印坐标或绘图显示
Console.WriteLine("Best match found at ({0}, {1})", rectangles[0].X, rectangles[0].Y);
image.DrawRectangle(rectangles[0], new Bgr(Color.Red), 2); // 绘制匹配区域
// 如果你想看到所有的匹配结果,可以遍历matches数组
foreach (var match in matches)
{
Console.WriteLine($"Score: {match.Score}, Location: ({match.Location.X}, {match.Location.Y})");
}
//
阅读全文
相关推荐
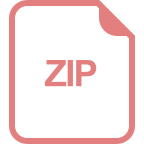
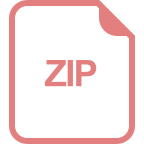
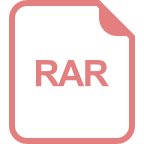


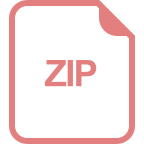
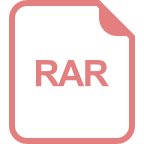
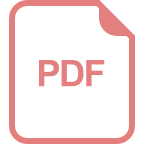
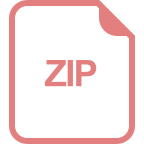





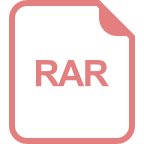
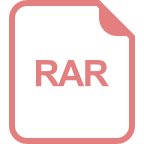
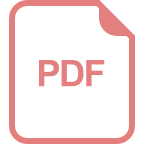

