二、实验内容(附源代码和运行结果的截图) 1.自建数据库,编写Mybatis核心配置文件,创建mybatis-config.xml文件,配置自己的数据库地址、名字、密码以及mysql驱动。 2.创建SqlSession完成基于配置的数据访问操作,完成基于注解的数据操作。 3.使用动态SQL语法,设计并实验一个多条件查询的功能模块。
时间: 2023-12-14 10:39:19 浏览: 174
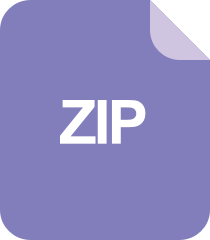
mybatis-3-config.dtd mybatis-3-mapper.dtd
1. 创建数据库
首先,我们需要在MySQL中创建一个数据库。以本次实验为例,我们创建一个名为`mybatis_demo`的数据库。
```sql
CREATE DATABASE mybatis_demo;
```
2. 编写Mybatis核心配置文件
在项目的classpath路径下创建一个名为`mybatis-config.xml`的文件,配置自己的数据库地址、名字、密码以及mysql驱动。
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis_demo"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
<mappers>
<!-- 在这里配置自己的Mapper文件 -->
</mappers>
</configuration>
```
3. 创建SqlSession完成基于配置的数据访问操作
在Mybatis中,我们需要通过SqlSession来执行SQL语句。SqlSession是线程不安全的,因此我们需要在每个线程中创建一个新的SqlSession对象。
```java
public class MybatisUtils {
private static SqlSessionFactory sqlSessionFactory;
static {
try {
// 加载Mybatis配置文件
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
// 构建SqlSessionFactory
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 获取SqlSession
*/
public static SqlSession getSqlSession() {
// 开启自动提交事务
return sqlSessionFactory.openSession(true);
}
}
```
在这个工具类中,我们通过`SqlSessionFactoryBuilder`来读取`mybatis-config.xml`文件,并构建一个`SqlSessionFactory`对象。然后,我们提供了一个`getSqlSession()`方法用于获取一个新的`SqlSession`对象。
4. 完成基于注解的数据操作
在Mybatis中,我们可以使用注解的方式来映射SQL语句。下面是一个例子:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM users WHERE id = #{id}")
User getUserById(int id);
@Insert("INSERT INTO users(username, password) VALUES(#{username}, #{password})")
int addUser(User user);
@Update("UPDATE users SET username = #{username}, password = #{password} WHERE id = #{id}")
int updateUser(User user);
@Delete("DELETE FROM users WHERE id = #{id}")
int deleteUser(int id);
}
```
在这个例子中,我们使用了`@Select`、`@Insert`、`@Update`、`@Delete`注解来定义了四个常用的数据库操作方法。其中,`#{}`语法表示SQL语句中的占位符。
5. 使用动态SQL语法,设计并实验一个多条件查询的功能模块
在Mybatis中,我们可以使用动态SQL语法来构建动态的SQL语句。下面是一个例子:
```java
@Mapper
public interface UserMapper {
List<User> getUsersByConditions(@Param("username") String username,
@Param("password") String password,
@Param("email") String email,
@Param("phone") String phone,
@Param("gender") String gender);
}
```
在这个例子中,我们使用了`@Param`注解来定义了五个参数,这些参数将用于构建动态SQL语句。下面是这个方法的具体实现:
```xml
<select id="getUsersByConditions" resultType="User">
SELECT * FROM users
<where>
<if test="username != null and username != ''">
AND username = #{username}
</if>
<if test="password != null and password != ''">
AND password = #{password}
</if>
<if test="email != null and email != ''">
AND email = #{email}
</if>
<if test="phone != null and phone != ''">
AND phone = #{phone}
</if>
<if test="gender != null and gender != ''">
AND gender = #{gender}
</if>
</where>
</select>
```
在这个动态SQL语句中,我们使用了`<where>`标签来包装多个`<if>`标签。每个`<if>`标签表示一个查询条件,它们将根据参数是否为null或空字符串来判断是否参与查询。最终,所有的查询条件将通过`AND`连接起来,构成一个完整的SQL语句。
下面是一个完整的测试代码:
```java
public class UserMapperTest {
private static UserMapper userMapper;
@BeforeAll
public static void setUp() {
// 获取SqlSession
SqlSession sqlSession = MybatisUtils.getSqlSession();
// 获取UserMapper代理对象
userMapper = sqlSession.getMapper(UserMapper.class);
}
@Test
public void testGetUsersByConditions() {
List<User> users = userMapper.getUsersByConditions("张三", null, null, null, null);
for (User user : users) {
System.out.println(user);
}
}
}
```
在这个测试代码中,我们获取了一个`SqlSession`对象并通过它获取了一个`UserMapper`代理对象。然后,我们调用了`getUsersByConditions()`方法进行了一次多条件查询,并输出了查询结果。
阅读全文
相关推荐
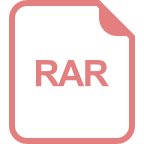

















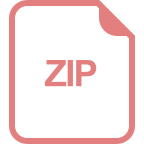