c语言编写程序实现:从键盘上输入一个年月日(以空格或回车分隔),求出该天是该年的第几天(注意判断该年是否是闰年)。输出格式为:sum days of 2012 -5 -30 :151
时间: 2024-10-29 17:25:14 浏览: 24
在C语言中,你可以通过以下步骤编写一个简单的程序来计算给定日期是一年中的第几天,同时判断该年是否是闰年:
```c
#include <stdio.h>
#include <stdbool.h>
bool is_leap_year(int year) {
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
return true;
}
return false;
}
int get_days_in_month(int month, int year) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default:
return 0; // Invalid month
}
}
int main() {
int year, month, day;
scanf("%d %d %d", &year, &month, &day);
// 检查输入合法性
if (month <= 0 || month > 12 || day <= 0 || day > get_days_in_month(month, year)) {
printf("Invalid date format.\n");
return 1;
}
int sum_days = 0;
for (int i = 1; i < month; ++i) {
sum_days += get_days_in_month(i, year);
}
sum_days += day;
printf("Sum days of %d-%02d-%02d : %d\n", year, month, day, sum_days);
return 0;
}
```
这个程序首先定义了一个`is_leap_year`函数来检查是否为闰年,然后计算每个月的天数,并在主函数中累加到总天数。最后,它会输出给定日期在该年中的总天数。
阅读全文
相关推荐
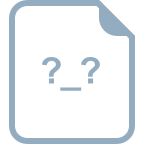
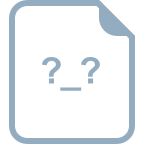
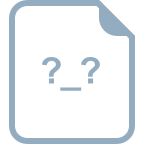
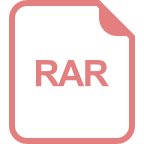
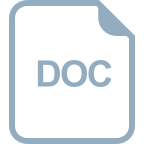
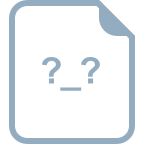
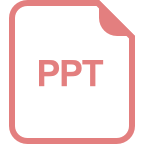
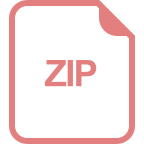
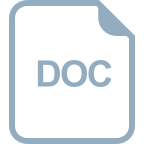
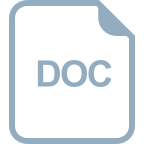
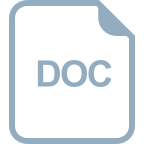
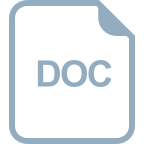
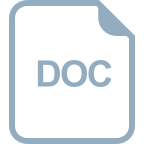