赋值运算符可以重载,但是无论参数为何种类型,赋值运算符都必须重载为成员函数,并且因为返回的是左值,所以返回值的类型必须是该类的
时间: 2024-05-09 22:20:46 浏览: 26
引用。重载赋值运算符的一般形式为:
```c++
class_name& operator=(const class_name& obj);
```
其中,`class_name`为类名,`obj`为要赋值的对象。在类的实现中,可以根据需要重载不同类型的赋值运算符,例如重载为接受常量指针的形式:
```c++
class_name& operator=(const class_name* obj);
```
在重载赋值运算符时,需要注意以下几点:
- 赋值运算符必须返回类的引用,以便支持链式赋值;
- 重载赋值运算符的参数类型必须与类的类型兼容,或者可以通过类型转换实现兼容;
- 赋值运算符应该检查自我赋值的情况,并且避免出现内存泄漏和资源浪费的问题;
- 赋值运算符应该尽量保持原有对象状态的不变性,避免出现不可预期的行为;
- 赋值运算符不应该抛出异常,否则可能导致程序异常终止。
下面是一个示例代码,演示了如何重载赋值运算符:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
public:
MyString() : str(nullptr), len(0) {}
MyString(const char* s) : str(nullptr), len(0) {
if (s) {
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
}
MyString(const MyString& other) : str(nullptr), len(0) {
if (other.str) {
len = other.len;
str = new char[len + 1];
strcpy(str, other.str);
}
}
~MyString() {
if (str) {
delete[] str;
str = nullptr;
len = 0;
}
}
MyString& operator=(const MyString& other) {
if (this != &other) {
if (str) {
delete[] str;
str = nullptr;
len = 0;
}
if (other.str) {
len = other.len;
str = new char[len + 1];
strcpy(str, other.str);
}
}
return *this;
}
const char* c_str() const { return str; }
private:
char* str;
size_t len;
};
int main() {
MyString s1("Hello");
MyString s2("World");
s1 = s2; // 调用重载的赋值运算符
cout << s1.c_str() << endl; // 输出 "World"
return 0;
}
```
上述代码中,`MyString`是一个简单的字符串类,支持动态分配内存和复制构造函数。在重载赋值运算符时,首先检查是否自我赋值,然后释放原有资源,再分配新的内存并复制数据。最后返回对象引用,以支持链式赋值。
相关推荐
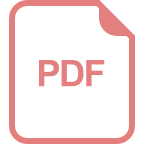
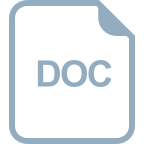
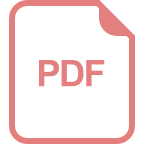














