写一个换装游戏代码带注释
时间: 2024-12-20 22:22:06 浏览: 6
编写一个简单的换装游戏代码示例,我们将使用Python语言和tkinter库来创建一个基础的界面。这个例子将包含人物、服装选择以及展示结果的部分。
```python
# 导入所需库
import tkinter as tk
from tkinter import ttk
import random
# 创建主窗口
class DressUpGame(tk.Tk):
def __init__(self):
super().__init__()
self.title("简易换装游戏")
self.geometry('400x600') # 设置窗口大小
# 定义人物角色
self.persons = ["公主", "王子", "忍者"]
self.selected_person = tk.StringVar() # 当前选择的人物
self.select_person_label = ttk.Label(self, textvariable=self.selected_person)
self.select_person_label.pack()
# 定义衣服选项
self.clothes = {
"裙子": {"颜色": ["粉色", "蓝色", "黄色"], "图案": ["花朵", "星星"]},
"上衣": {"颜色": ["白色", "黑色", "灰色"], "款式": ["衬衫", "毛衣"]}
}
# 选择衣服部分
self.choice_frame = ttk.Frame(self)
self.choice_frame.pack()
for category, options in self.clothes.items():
clothes_list = ttk.Combobox(self.choice_frame, values=options.keys())
clothes_list.current(0) # 设置默认值
clothes_list.bind("<<ComboboxSelected>>", lambda event: self.update_dress(clothes_list, category))
clothes_list.grid(row=0, column=tk.grid_columnconfigure(0, weight=1), padx=10)
# 展示结果部分
self.result_label = ttk.Label(self, text="装扮结果:")
self.result_label.pack()
def update_dress(self, combobox, category):
selected_cloth = combobox.get()
color_options = self.clothes[category]["颜色"]
pattern_options = self.clothes[category]["图案"]
# 随机选取服装属性
color = random.choice(color_options)
if category == "裙子":
pattern = random.choice(pattern_options)
else:
pattern = None
# 更新结果显示
result_text = f"{self.selected_person.get()} 穿着 {selected_cloth},{color}{', ' + ('有' if pattern else '') + pattern}"
self.result_label.config(text=result_text)
if __name__ == "__main__":
game = DressUpGame()
game.mainloop()
```
在这个代码中,用户可以选择人物和衣物类别,程序会随机从预设的颜色和图案中挑选出一种组合来显示结果。你可以根据需求进一步扩展服装选项和增加更多的交互功能。
阅读全文
相关推荐
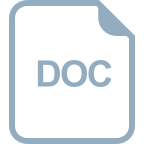
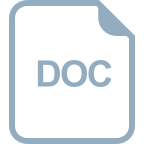
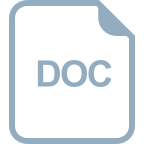

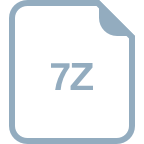
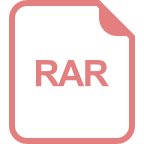
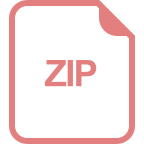
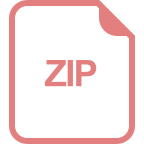
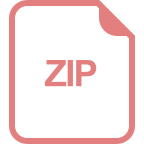
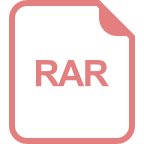


