写一个换装代码带图片标注和注释
时间: 2024-12-20 21:15:47 浏览: 11
在Python中编写一个简单的换装小游戏,我们这里可以使用pygame库来创建一个界面,并添加一些基础的图像换装元素。以下是简化的示例代码和注释:
```python
# 导入必要的模块
import pygame
from pygame.locals import *
# 初始化pygame
pygame.init()
# 设置窗口大小
window_size = (600, 400)
screen = pygame.display.set_mode(window_size)
# 图片加载
# 这里假设我们有两个衣服图片,如"shirt.png"和"pants.png"
shirt_img = pygame.image.load('shirt.png')
pants_img = pygame.image.load('pants.png')
# 定义人物和衣物位置
player_x, player_y = 50, 50
shirt_rect = shirt_img.get_rect(center=(player_x, player_y))
pants_rect = pants_img.get_rect(center=(player_x, player_y + shirt_img.get_height()))
# 主游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
running = False
# 更新屏幕内容
screen.fill((0, 0, 0)) # 渲染黑色背景
screen.blit(shirt_img, shirt_rect) # 绘制上衣
screen.blit(pants_img, pants_rect) # 绘制裤子
pygame.draw.circle(screen, (255, 255, 255), (player_x, player_y), 10) # 绘制人物头部作为标记
# 更新显示
pygame.display.flip()
# 结束游戏
pygame.quit()
```
**注释说明:**
1. 首先导入了pygame库并初始化它。
2. 确定游戏窗口尺寸,并设置一个主屏幕。
3. 加载衣服图片,并通过get_rect方法获取矩形区域,方便后续的位置调整。
4. 游戏主循环,处理用户退出请求和其他事件。
5. 清空屏幕、绘制上衣、裤子,并在人物头部处画一个小白点作为标记。
6. 每次循环都更新屏幕显示。
7. 最后关闭pygame。
**
阅读全文
相关推荐
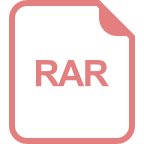
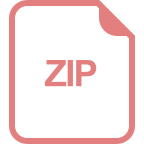

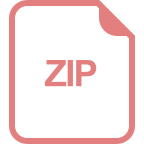
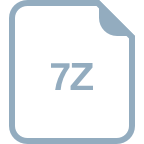
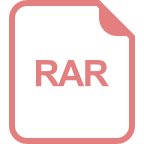
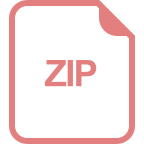
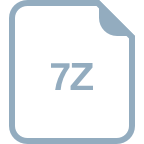
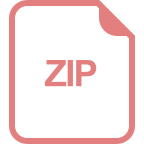
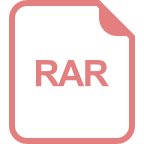







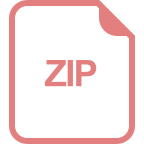