如何将C语言中的集合数据结构转换为数组表示?
时间: 2024-10-05 16:01:33 浏览: 15
在C语言中,虽然原生并没有内置的集合数据结构,但我们通常会自定义实现,比如链表、哈希表等。如果你想要将这些自定义的集合转换为数组表示,可以采取以下步骤:
1. **选择合适的数据结构**:首先确定你的集合是否已经是动态数组(如链表),如果是动态数组,直接就是数组形式。
2. **遍历集合**:对于其他非数组型的集合(如链表、树等),需要通过迭代或递归的方式遍历整个集合,记录每个元素的值。
3. **创建新数组**:根据遍历过程中元素的数量,动态分配一个新的固定大小的数组。
4. **复制元素**:将每个遍历到的元素添加到新数组中对应的位置。
5. **初始化新数组**:如果数组未满,填充剩余位置(这一步可以根据实际需求选择是否做)。
例如,假设有一个链表,你可以这样做:
```c
struct Node {
int value;
struct Node* next;
};
// 转换函数
int* collection_to_array(Node* head) {
int count = 0;
Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
int* array = malloc(count * sizeof(int));
current = head;
for (int i = 0; i < count; i++) {
array[i] = current->value;
current = current->next;
}
return array;
}
```
相关推荐
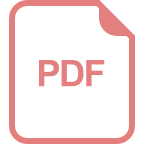
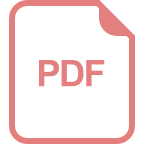















