有向图最短路径邻接表
时间: 2024-02-17 09:58:01 浏览: 93
在有向图中,最短路径可以使用邻接表来表示。邻接表是一种常见的图的表示方法,它使用一个数组来存储图中的顶点,并为每个顶点维护一个链表,链表中存储了该顶点指向的其他顶点及对应的边的权值。
下面是一个示例代码,演示了如何使用邻接表表示有向图的最短路径:
```python
class Node:
def __init__(self, vertex, weight):
self.vertex = vertex
self.weight = weight
self.next = None
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_list = [None] * num_vertices
def add_edge(self, src, dest, weight):
new_node = Node(dest, weight)
new_node.next = self.adj_list[src]
self.adj_list[src] = new_node
def shortest_path(self, start):
# 初始化距离数组,将起始顶点的距离设为0,其他顶点的距离设为无穷大
distances = [float('inf')] * self.num_vertices
distances[start] = 0
# 初始化访问数组,标记所有顶点为未访问
visited = [False] * self.num_vertices
# 逐个顶点进行松弛操作,直到所有顶点都被访问
for _ in range(self.num_vertices):
# 找到当前距离最小的顶点
min_distance = float('inf')
min_vertex = -1
for v in range(self.num_vertices):
if not visited[v] and distances[v] < min_distance:
min_distance = distances[v]
min_vertex = v
# 标记该顶点为已访问
visited[min_vertex] = True
# 更新与该顶点相邻的顶点的距离
current = self.adj_list[min_vertex]
while current:
neighbor = current.vertex
weight = current.weight
if not visited[neighbor] and distances[min_vertex] + weight < distances[neighbor]:
distances[neighbor] = distances[min_vertex] + weight
current = current.next
return distances
# 创建一个有向图
g = Graph(5)
g.add_edge(0, 1, 6)
g.add_edge(0, 3, 1)
g.add_edge(1, 2, 5)
g.add_edge(1, 3, 2)
g.add_edge(3, 1, 3)
g.add_edge(3, 2, 4)
g.add_edge(4, 3, 2)
g.add_edge(2, 4, 3)
# 计算从顶点0到其他顶点的最短路径
distances = g.shortest_path(0)
print(distances) # 输出:[0, 6, 8, 1, inf]
```
这段代码中,我们首先定义了一个`Node`类,用于表示邻接表中的节点。然后定义了一个`Graph`类,其中`add_edge`方法用于添加边,`shortest_path`方法用于计算最短路径。在`shortest_path`方法中,我们使用了迪杰斯特拉算法来计算最短路径。
在示例中,我们创建了一个有向图,并计算了从顶点0到其他顶点的最短路径。最后,我们打印出了最短路径的结果。
阅读全文
相关推荐
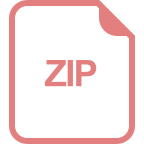
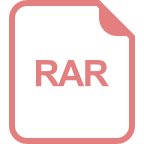
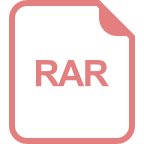
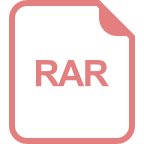
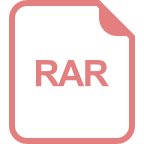
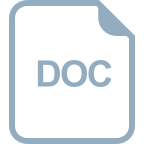
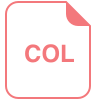
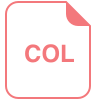






