springmvc实现批量删除
时间: 2024-07-04 10:00:50 浏览: 83
在Spring MVC中实现批量删除功能,通常涉及到以下几个步骤:
1. 创建一个控制器方法:这个方法接收一个包含多个ID的请求参数,例如一个JSON数组或是一个逗号分隔的字符串。
```java
@PostMapping("/batchDelete")
public ResponseEntity<?> deleteBatch(@RequestBody List<Integer> ids) {
// Your code here
}
```
2. 配置数据访问层:使用JPA或HQL等ORM技术,编写一个Service或Repository方法,该方法接受一个整数列表,然后根据这些ID从数据库中删除相应的记录。
```java
@Service
public class YourService {
@Autowired
private YourRepository repository;
public void deleteBatch(List<Integer> ids) {
ids.forEach(id -> repository.deleteById(id));
}
}
```
3. 数据验证:在Controller层检查输入的ID是否有效,如果无效则返回错误信息。
```java
public ResponseEntity<?> deleteBatch(@Valid List<Integer> ids, BindingResult result) {
if (result.hasErrors()) {
return ResponseEntity.badRequest().body(result.getAllErrors());
}
// Continue with the deletion process
}
```
4. 异常处理:添加异常处理代码,如可能出现的数据库连接错误、事务回滚等。
5. 返回响应:删除操作完成后,可以选择返回一个确认信息或者直接返回HTTP状态码,比如204表示成功删除,400表示请求无效。
```java
@PostMapping("/batchDelete")
public ResponseEntity<?> deleteBatch(@Valid List<Integer> ids, BindingResult result) {
if (result.hasErrors()) {
return ResponseEntity.badRequest().body(result.getAllErrors());
}
try {
yourService.deleteBatch(ids);
return ResponseEntity.noContent().build();
} catch (Exception e) {
log.error("Error deleting batch", e);
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
```
相关推荐
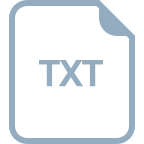
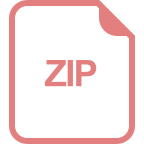
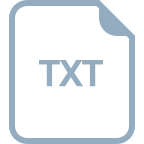
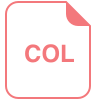
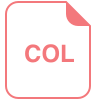
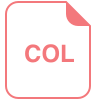
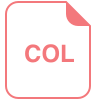
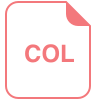









