STM32 ADC 采样 正弦波 代码
时间: 2024-02-09 08:41:51 浏览: 34
下面是一个基于STM32的ADC采集正弦波的示例代码:
首先,需要配置ADC模块的参数,包括采样通道、采样时间、采样时钟等。在此示例中,我们使用PA0引脚作为ADC采样通道,采样时间为15个时钟周期,采样时钟为PCLK2/2。
```
// Configure ADC
ADC_InitTypeDef ADC_InitStruct;
ADC_CommonInitTypeDef ADC_CommonInitStruct;
ADC_CommonInitStruct.ADC_Mode = ADC_Mode_Independent;
ADC_CommonInitStruct.ADC_Prescaler = ADC_Prescaler_Div2;
ADC_CommonInitStruct.ADC_DMAAccessMode = ADC_DMAAccessMode_Disabled;
ADC_CommonInitStruct.ADC_TwoSamplingDelay = ADC_TwoSamplingDelay_5Cycles;
ADC_CommonInit(&ADC_CommonInitStruct);
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = ENABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_ExternalTrigConv = ADC_ExternalTrigConv_T1_CC1;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_15Cycles);
ADC_Cmd(ADC1, ENABLE);
```
接下来,我们可以使用TIM2定时器来生成正弦波信号。在此示例中,我们使用PA1引脚输出正弦波信号,TIM2定时器的周期为1000个时钟周期,即1kHz的频率。为了产生正弦波,我们使用一个256个点的正弦波表,每个点的值为-1到1之间的浮点数。
```
// Configure TIM2
TIM_TimeBaseInitTypeDef TIM_InitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_1;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource1, GPIO_AF_TIM2);
TIM_TimeBaseStructInit(&TIM_InitStruct);
TIM_InitStruct.TIM_Period = 1000;
TIM_InitStruct.TIM_Prescaler = 84;
TIM_InitStruct.TIM_ClockDivision = 0;
TIM_InitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_InitStruct);
TIM_Cmd(TIM2, ENABLE);
// Generate sine wave
float sine_table[256] = {0.0000, 0.0245, 0.0491, 0.0736, 0.0980, 0.1224, 0.1467, 0.1710, 0.1951, 0.2191, 0.2429, 0.2667, 0.2903, 0.3137, 0.3369, 0.3599, 0.3827, 0.4052, 0.4276, 0.4496, 0.4714, 0.4929, 0.5141, 0.5350, 0.5556, 0.5758, 0.5957, 0.6152, 0.6344, 0.6532, 0.6716, 0.6895, 0.7071, 0.7242, 0.7409, 0.7572, 0.7730, 0.7883, 0.8032, 0.8176, 0.8315, 0.8449, 0.8577, 0.8701, 0.8819, 0.8932, 0.9039, 0.9142, 0.9239, 0.9330, 0.9415, 0.9495, 0.9569, 0.9638, 0.9700, 0.9757, 0.9808, 0.9853, 0.9892, 0.9925, 0.9952, 0.9973, 0.9988, 0.9997, 1.0000, 0.9997, 0.9988, 0.9973, 0.9952, 0.9925, 0.9892, 0.9853, 0.9808, 0.9757, 0.9700, 0.9638, 0.9569, 0.9495, 0.9415, 0.9330, 0.9239, 0.9142, 0.9039, 0.8932, 0.8819, 0.8701, 0.8577, 0.8449, 0.8315, 0.8176, 0.8032, 0.7883, 0.7730, 0.7572, 0.7409, 0.7242, 0.7071, 0.6895, 0.6716, 0.6532, 0.6344, 0.6152, 0.5957, 0.5758, 0.5556, 0.5350, 0.5141, 0.4929, 0.4714, 0.4496, 0.4276, 0.4052, 0.3827, 0.3599, 0.3369, 0.3137, 0.2903, 0.2667, 0.2429, 0.2191, 0.1951, 0.1710, 0.1467, 0.1224, 0.0980, 0.0736, 0.0491, 0.0245, 0.0000, -0.0245, -0.0491, -0.0736, -0.0980, -0.1224, -0.1467, -0.1710, -0.1951, -0.2191, -0.2429, -0.2667, -0.2903, -0.3137, -0.3369, -0.3599, -0.3827, -0.4052, -0.4276, -0.4496, -0.4714, -0.4929, -0.5141, -0.5350, -0.5556, -0.5758, -0.5957, -0.6152, -0.6344, -0.6532, -0.6716, -0.6895, -0.7071, -0.7242, -0.7409, -0.7572, -0.7730, -0.7883, -0.8032, -0.8176, -0.8315, -0.8449, -0.8577, -0.8701, -0.8819, -0.8932, -0.9039, -0.9142, -0.9239, -0.9330, -0.9415, -0.9495, -0.9569, -0.9638, -0.9700, -0.9757, -0.9808, -0.9853, -0.9892, -0.9925, -0.9952, -0.9973, -0.9988, -0.9997, -1.0000, -0.9997, -0.9988, -0.9973, -0.9952, -0.9925, -0.9892, -0.9853, -0.9808, -0.9757, -0.9700, -0.9638, -0.9569, -0.9495, -0.9415, -0.9330, -0.9239, -0.9142, -0.9039, -0.8932, -0.8819, -0.8701, -0.8577, -0.8449, -0.8315, -0.8176, -0.8032, -0.7883, -0.7730, -0.7572, -0.7409, -0.7242, -0.7071, -0.6895, -0.6716, -0.6532, -0.6344, -0.6152, -0.5957, -0.5758, -0.5556, -0.5350, -0.5141, -0.4929, -0.4714, -0.4496, -0.4276, -0.4052, -0.3827, -0.3599, -0.3369, -0.3137, -0.2903, -0.2667, -0.2429, -0.2191, -0.1951, -0.1710, -0.1467, -0.1224, -0.0980, -0.0736, -0.0491, -0.0245};
uint32_t sine_index = 0;
while (1)
{
// Set duty cycle of TIM2 to generate sine wave
uint16_t duty_cycle = (sine_table[sine_index] + 1) * 500;
TIM2->CCR1 = duty_cycle;
// Start ADC conversion
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
// Get ADC value and convert to voltage
uint16_t adc_value = ADC_GetConversionValue(ADC1);
float voltage = adc_value * 3.3 / 4096;
// Increment sine wave index and wrap around at 256
sine_index++;
if (sine_index >= 256)
sine_index = 0;
}
```
在主循环中,我们不断地更新TIM2的占空比来输出正弦波信号,并且在每个周期开始时启动ADC转换。一旦转换完成,我们可以获取ADC值并将其转换为电压值,然后可以对电压值进行进一步处理或显示。
相关推荐
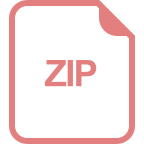
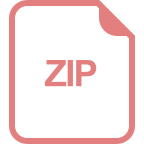














