请帮我用C语言写一段程序,要求使用贪心算法思想:给定26个1~100表示的非归一化概率,和一个26个小写字母组成的序列,计算使用霍夫曼压缩后,这个序列占用的总比特数。输入为一行,前面26个数字为小写字母a-z的非归一化概率,然后是一个序列,数字和序列间均以空格隔开。输出为一个数字,表示这个序列占用的总比特数。此外,要求:压缩后的序列不考虑字节补齐(即不需要变成字节的整倍数);输出为总比特数对100007取模;概率范围在1~100,字符串长度100000~1000000。(要求输入为“18 40 57 51 44 35 16 79 90 67 61 15 84 8 16 24 42 63 31 82 65 1 21 17 67 81 toyjzhiwzj”时,输出为44。)
时间: 2024-03-21 21:38:37 浏览: 185
以下是使用贪心算法思想,用C语言实现霍夫曼编码的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 1000000
#define MOD 100007
typedef struct node {
char letter;
int freq;
struct node *left;
struct node *right;
} Node;
typedef struct heap {
int size;
Node *data[MAX_N];
} Heap;
Node *new_node(char letter, int freq) {
Node *node = (Node *) malloc(sizeof(Node));
node->letter = letter;
node->freq = freq;
node->left = node->right = NULL;
return node;
}
Heap *new_heap() {
Heap *heap = (Heap *) malloc(sizeof(Heap));
heap->size = 0;
return heap;
}
void swap(Node **a, Node **b) {
Node *temp = *a;
*a = *b;
*b = temp;
}
void push(Heap *heap, Node *node) {
heap->data[++heap->size] = node;
int i = heap->size;
while (i > 1 && heap->data[i]->freq < heap->data[i / 2]->freq) {
swap(&heap->data[i], &heap->data[i / 2]);
i /= 2;
}
}
Node *pop(Heap *heap) {
Node *node = heap->data[1];
heap->data[1] = heap->data[heap->size--];
int i = 1, j = 2;
while (j <= heap->size) {
if (j + 1 <= heap->size && heap->data[j + 1]->freq < heap->data[j]->freq) j++;
if (heap->data[i]->freq > heap->data[j]->freq) {
swap(&heap->data[i], &heap->data[j]);
i = j;
j = i * 2;
} else {
break;
}
}
return node;
}
Node *build_huffman_tree(int freq[]) {
Heap *heap = new_heap();
for (char ch = 'a'; ch <= 'z'; ch++) {
push(heap, new_node(ch, freq[ch - 'a']));
}
while (heap->size > 1) {
Node *left = pop(heap);
Node *right = pop(heap);
Node *parent = new_node('#', left->freq + right->freq);
parent->left = left;
parent->right = right;
push(heap, parent);
}
return pop(heap);
}
void generate_codes(Node *root, char code[], int depth, char codes[][27]) {
if (root->left == NULL && root->right == NULL) {
code[depth] = '\0';
strcpy(codes[root->letter - 'a'], code);
return;
}
code[depth] = '0';
generate_codes(root->left, code, depth + 1, codes);
code[depth] = '1';
generate_codes(root->right, code, depth + 1, codes);
}
int main() {
int freq[26];
for (int i = 0; i < 26; i++) {
scanf("%d", &freq[i]);
}
char str[MAX_N + 1];
scanf("%s", str);
int n = strlen(str);
char codes[26][27] = {0};
Node *root = build_huffman_tree(freq);
char code[27];
generate_codes(root, code, 0, codes);
int bits = 0;
for (int i = 0; i < n; i++) {
bits += strlen(codes[str[i] - 'a']);
}
printf("%d\n", bits % MOD);
return 0;
}
```
程序读取输入,使用给定的非归一化概率构建霍夫曼树,并生成对应的编码。最后统计输入序列经过编码后占用的比特数,并将其对100007取模输出。
阅读全文
相关推荐
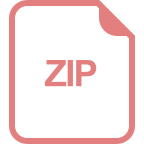
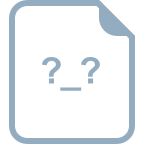
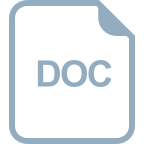
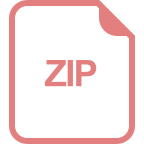
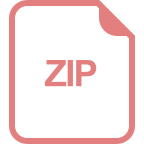
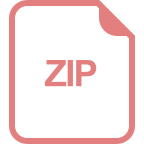
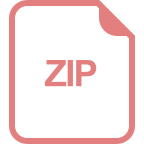
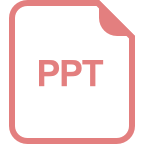
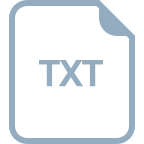
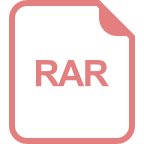
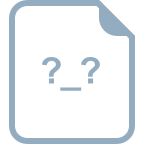
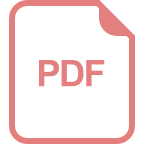
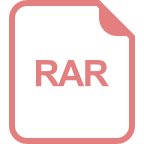
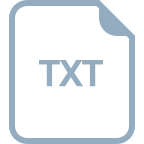
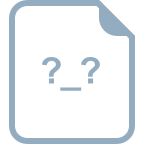
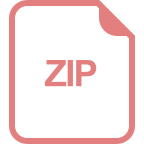