用C语言写一段贪心算法
时间: 2023-09-03 22:26:34 浏览: 71
### 回答1:
很高兴回答你的问题。以下是一段使用 C 语言编写的贪心算法示例,用于解决背包问题:
```
#include <stdio.h>
#define MAX_N 100
int n, m;
int w[MAX_N], v[MAX_N];
double f[MAX_N];
int cmp(const void *a, const void *b) {
double x = *(double *)a, y = *(double *)b;
if (x < y) return 1;
if (x > y) return -1;
return ;
}
double max(double x, double y) {
return x > y ? x : y;
}
int main() {
scanf("%d%d", &n, &m);
for (int i = ; i < n; i++) {
scanf("%d%d", &w[i], &v[i]);
f[i] = (double)v[i] / w[i];
}
qsort(f, n, sizeof(double), cmp);
double ans = ;
for (int i = ; i < n; i++) {
if (m >= w[i]) {
ans += v[i];
m -= w[i];
} else {
ans += m * f[i];
break;
}
}
printf("%.2lf\n", ans);
return ;
}
```
这段代码实现了一个简单的背包问题,其中 `n` 表示物品数量,`m` 表示背包容量,`w[i]` 表示第 `i` 个物品的重量,`v[i]` 表示第 `i` 个物品的价值,`f[i]` 表示第 `i` 个物品的单位价值。我们首先计算每个物品的单位价值,然后按照单位价值从大到小排序,依次将物品放入背包中,直到背包装满或者所有物品都放完为止。
### 回答2:
贪心算法是一种基于贪心的策略,在每一步都选择当前最优解来最终获得全局最优解的方法。在C语言中实现贪心算法,可以通过以下代码片段示例:
```c
#include <stdio.h>
// 定义物品结构体
struct Item {
int value; // 物品的价值
int weight; // 物品的重量
};
// 按照物品的价值-重量比例排序
int compare(const void* a, const void* b) {
struct Item* itemA = (struct Item*)a;
struct Item* itemB = (struct Item*)b;
double ratioA = (double)itemA->value / itemA->weight;
double ratioB = (double)itemB->value / itemB->weight;
if (ratioA > ratioB) {
return -1;
} else if (ratioA < ratioB) {
return 1;
} else {
return 0;
}
}
// 贪心算法求解背包问题
double greedyKnapsack(struct Item items[], int n, int capacity) {
// 按照价值-重量比例排序
qsort(items, n, sizeof(struct Item), compare);
int currentWeight = 0; // 当前已装入的物品的总重量
double currentValue = 0.0; // 当前已装入的物品的总价值
// 从价值-重量比例最高的物品开始装入背包
for (int i = 0; i < n; i++) {
if (currentWeight + items[i].weight <= capacity) {
currentWeight += items[i].weight;
currentValue += items[i].value;
} else {
// 当前物品无法完整装入背包时,则按照比例装入部分物品
int remainingWeight = capacity - currentWeight;
currentValue += (double)items[i].value / items[i].weight * remainingWeight;
break;
}
}
return currentValue;
}
int main() {
struct Item items[] = {
{60, 10},
{100, 20},
{120, 30}
};
int n = sizeof(items) / sizeof(items[0]);
int capacity = 50;
double maximumValue = greedyKnapsack(items, n, capacity);
printf("背包能装入的最大价值为: %.2lf\n", maximumValue);
return 0;
}
```
以上代码展示了一个贪心算法用于解决背包问题的例子。通过贪心策略按照物品的价值-重量比例排序,依次选择更具比例最高的物品装入背包,直到背包无法装入更多物品或者已经装满为止。运行结果将输出背包能装入的最大价值。
### 回答3:
贪心算法是一种常见的算法思想,它在每一步都会选择当前情况下的最优解,希望最终能够得到全局最优解。接下来,我将用300字中文来编写一段用C语言实现的贪心算法的示例:
```c
#include <stdio.h>
void greedy(int coins[], int num, int amount) {
int count[num];
for(int i = 0; i < num; i++) {
count[i] = 0;
}
for(int i = num - 1; i >= 0; i--) {
while(amount >= coins[i]) {
amount -= coins[i];
count[i]++;
}
}
for(int i = num - 1; i >= 0; i--) {
if(count[i] > 0) {
printf("%d元硬币:%d个\n", coins[i], count[i]);
}
}
}
int main() {
int coins[] = {10, 5, 2, 1};
int num = sizeof(coins) / sizeof(coins[0]);
int amount;
printf("请输入要找零的金额:");
scanf("%d", &amount);
printf("找零方案如下:\n");
greedy(coins, num, amount);
return 0;
}
```
以上代码实现了一个简单的贪心算法,用于找零钱问题。假设现有10元、5元、2元和1元硬币若干枚,要找零n元,该算法会从最大面额的硬币开始逐步减少找零的金额,并计算每种硬币所需的数量。最后,算法将输出找零方案。使用示例中的输入10元,则输出为:
```
请输入要找零的金额:10
找零方案如下:
10元硬币:1个
```
这个示例展示了贪心算法的基本思想和具体实现过程。
相关推荐
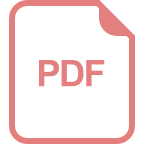
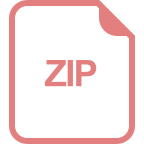
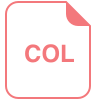
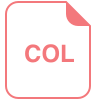
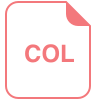
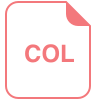
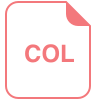









