在c++中输入一个字符串再输入一个字符输出该字符串在字符串中所对应的最大下标(下标从0开始),或者否则输出“Not Found”
时间: 2023-05-22 09:03:51 浏览: 49
可以使用strchr函数获取字符串中某个字符的指针,然后用指针减去字符串的首地址,即可得到该字符在字符串中的下标。如果该字符不在字符串中,则返回NULL。下面是示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
char c;
printf("请输入字符串:");
fgets(str, sizeof(str), stdin);
printf("请输入字符:");
scanf("%c", &c);
char* p = strchr(str, c);
if (p != NULL) {
printf("最大下标为:%d\n", p - str - 1);
} else {
printf("Not Found\n");
}
return 0;
}
```
注意:fgets函数会读取一整行,包括回车符,因此计算下标时需要减去1。
相关问题
c++输入一个字符串,再输入一个字符在字符串中删除字符形成新的字符串
可以使用C++的STL库中的字符串类string来实现。
具体步骤如下:
1. 输入原始字符串和要删除的字符,可以使用cin或getline函数。
2. 使用string类的find函数查找要删除的字符在字符串中的位置,如果找到了,则返回该字符在字符串中的下标;如果没找到,则返回string::npos。
3. 如果find函数返回的不是string::npos,则说明找到了要删除的字符,可以使用string类的erase函数将该字符从字符串中删除。
4. 最后输出删除后的字符串即可。
示例代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str;
char ch;
// 输入原始字符串和要删除的字符
cout << "请输入一个字符串:";
getline(cin, str);
cout << "请输入要删除的字符:";
cin >> ch;
// 查找要删除的字符在字符串中的位置
size_t pos = str.find(ch);
// 删除字符并输出结果
if (pos != string::npos) {
str.erase(pos, 1);
cout << "删除后的字符串为:" << str << endl;
} else {
cout << "字符串中不存在该字符!" << endl;
}
return 0;
}
```
运行结果:
```
请输入一个字符串:hello world
请输入要删除的字符:o
删除后的字符串为:hell wrld
```
输入一个字符串数组和一个字符查找并输出字符在字符串数组中首次出现的位置用c++
以下是一个示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string arr[] = {"hello", "world", "test", "code"};
char target = 'e';
int index = -1;
// 遍历字符串数组,查找目标字符
for (int i = 0; i < sizeof(arr) / sizeof(arr[0]); i++) {
if (arr[i].find(target) != string::npos) {
index = i;
break;
}
}
// 输出结果
if (index == -1) {
cout << "Target character not found in array." << endl;
} else {
cout << "Target character found at index " << index << " in array." << endl;
}
return 0;
}
```
在上述代码中,我们首先定义了一个字符串数组 `arr` 和一个目标字符 `target`。然后我们遍历整个数组,对于每个字符串都调用 `find` 函数查找目标字符。如果找到了目标字符,我们就记录下当前字符串在数组中的下标。最后根据记录的下标输出结果。如果目标字符没有出现在数组中,我们就输出一个提示信息。
相关推荐
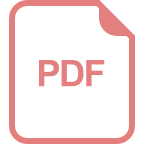












