python websocket 与 unity websocket通讯
时间: 2023-06-27 20:04:00 浏览: 304
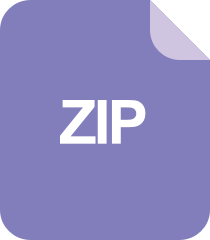
unity和python通过自定义socket协议实现异步通信
要在Python和Unity之间进行WebSocket通信,您需要使用一个WebSocket库来在Python中建立WebSocket服务器,并使用Unity的WebSocket API来连接该服务器并发送/接收消息。
以下是一个简单的示例,演示如何使用Python Tornado库作为WebSocket服务器,以及如何在Unity中使用WebSocket API连接该服务器:
Python服务器:
```python
import tornado.ioloop
import tornado.web
import tornado.websocket
class WebSocketHandler(tornado.websocket.WebSocketHandler):
def open(self):
print("WebSocket opened")
def on_message(self, message):
print("Received message: " + message)
self.write_message("You said: " + message)
def on_close(self):
print("WebSocket closed")
app = tornado.web.Application([
(r"/websocket", WebSocketHandler),
])
if __name__ == "__main__":
app.listen(8888)
print("WebSocket server started")
tornado.ioloop.IOLoop.instance().start()
```
Unity客户端:
```csharp
using UnityEngine;
using WebSocketSharp;
public class WebSocketClient : MonoBehaviour
{
private WebSocket ws;
void Start()
{
ws = new WebSocket("ws://localhost:8888/websocket");
ws.OnOpen += OnOpen;
ws.OnMessage += OnMessage;
ws.OnClose += OnClose;
ws.Connect();
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
ws.Send("Hello from Unity!");
}
}
void OnOpen(object sender, System.EventArgs e)
{
Debug.Log("WebSocket opened");
}
void OnMessage(object sender, MessageEventArgs e)
{
Debug.Log("Received message: " + e.Data);
}
void OnClose(object sender, CloseEventArgs e)
{
Debug.Log("WebSocket closed");
}
}
```
您需要确保Python服务器和Unity客户端都在同一网络中,并且您需要将Python服务器的IP地址和端口号更新到Unity客户端的WebSocket连接中。
阅读全文
相关推荐
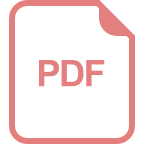



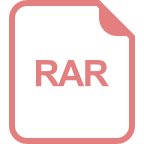
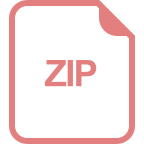
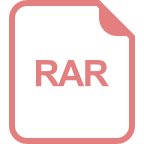
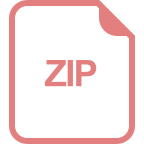
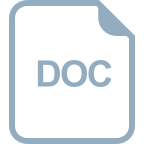
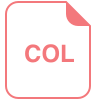
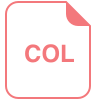
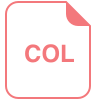
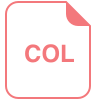
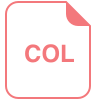
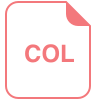
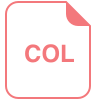