按照以下要求设计接口IArea、长方形类Rectangle、正方形类Square、圆形类 Circle,最终在主函数中输出长方形、正方形及圆形面积. 1.接口IArea,包含:计算面积的方法doubleArea(); 2.类Rectangle、类Square、类Circle继承接口,包含: ①自动属性; ②构造函数(能判断对应参数的合理性(如:x>0)); ③实现计算面积的方法Area(); 3.主函数中,创建三个对象(如下:),分别调用方法Area(),输出三种图形的面积。 TArea r= new Rectangle(3, 5); /Rectangle r = new Rectangle(3, 5) Console.WriteLine(”长方形的面积为:{0:0.00}”,r.Area0): PO.VI s =new Square(3): Console.WriteLine(”正方形的面积为:{0:0.00}”,s.Area() TArea c = new Circle(2): Console.WriteLine(“圆形的面积为:{0:0.00}”,c.Area()); 输出样例: 方形的面积为:15.00 正方形的面积为:9.00 圆形的面积为:12.57使用cSHARP编写
时间: 2023-12-06 14:44:43 浏览: 214
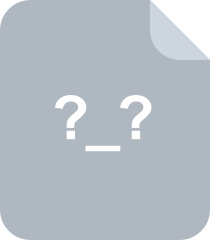
设计基类点类(Point)、直接派生类圆类(Circle)、间接派生类圆柱体类(Cylinder),将求面积的函数(area)声明成虚函数,并在主函数实现虚函数
以下是使用C#编写的代码:
```csharp
using System;
interface IArea
{
double Area();
}
class Rectangle : IArea
{
private double length;
private double width;
public Rectangle(double l, double w)
{
if (l > 0 && w > 0)
{
length = l;
width = w;
}
else
{
throw new Exception("Length and width must be greater than 0!");
}
}
public double Area()
{
return length * width;
}
}
class Square : IArea
{
private double side;
public Square(double s)
{
if (s > 0)
{
side = s;
}
else
{
throw new Exception("Side length must be greater than 0!");
}
}
public double Area()
{
return side * side;
}
}
class Circle : IArea
{
private double radius;
public Circle(double r)
{
if (r > 0)
{
radius = r;
}
else
{
throw new Exception("Radius must be greater than 0!");
}
}
public double Area()
{
return Math.PI * radius * radius;
}
}
class Program
{
static void Main(string[] args)
{
IArea r = new Rectangle(3, 5);
Console.WriteLine("长方形的面积为:{0:0.00}", r.Area());
IArea s = new Square(3);
Console.WriteLine("正方形的面积为:{0:0.00}", s.Area());
IArea c = new Circle(2);
Console.WriteLine("圆形的面积为:{0:0.00}", c.Area());
}
}
```
输出结果为:
```
长方形的面积为:15.00
正方形的面积为:9.00
圆形的面积为:12.57
```
阅读全文
相关推荐
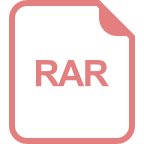


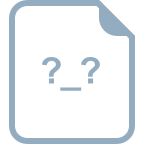
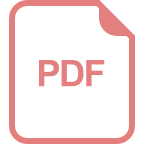
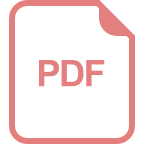
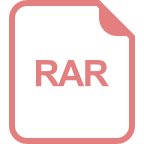
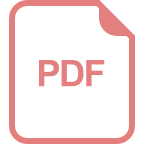
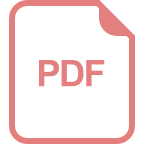
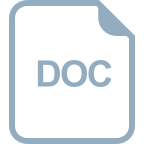
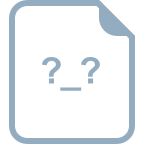
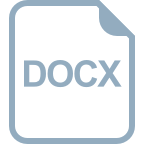
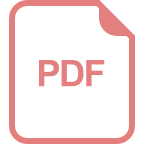
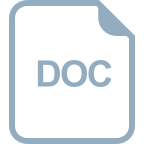
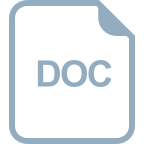
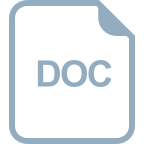
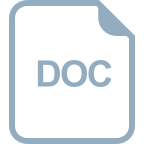