用Cshape 按照以下要求设计接口lArea、长方形类Rectangle、正方形类Square、圆形类Circle,最终在主函数中输出长方形、正方形及圆形面积。 1.接口IArea,包含:计算面积的方法double Area(); 2.类Rectangle、类Square、类Circle 继承接口,包含: ①自动属性; ②构造函数(能判断对应参数的合理性(如:x>0)); ③实现计算面积的方法Area(); 3. 主函数中,创建三个对象(如下: ), 分别调用方法Area(), 输出三种图形的面积。
时间: 2023-12-06 21:45:32 浏览: 147
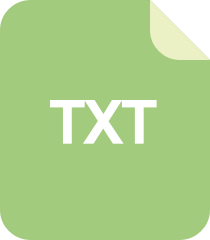
设计一个Shape接口和它的两个实现类Square和Circle,要求如下
以下是代码示例:
```csharp
using System;
// 定义接口
interface IArea {
double Area();
}
// 定义长方形类
class Rectangle : IArea {
// 自动属性
public double Width { get; }
public double Height { get; }
// 构造函数
public Rectangle(double width, double height) {
if (width <= 0 || height <= 0) {
throw new ArgumentException("长方形的宽和高必须大于0");
}
Width = width;
Height = height;
}
// 实现计算面积的方法
public double Area() {
return Width * Height;
}
}
// 定义正方形类
class Square : IArea {
// 自动属性
public double Side { get; }
// 构造函数
public Square(double side) {
if (side <= 0) {
throw new ArgumentException("正方形的边长必须大于0");
}
Side = side;
}
// 实现计算面积的方法
public double Area() {
return Side * Side;
}
}
// 定义圆形类
class Circle : IArea {
// 自动属性
public double Radius { get; }
// 构造函数
public Circle(double radius) {
if (radius <= 0) {
throw new ArgumentException("圆的半径必须大于0");
}
Radius = radius;
}
// 实现计算面积的方法
public double Area() {
return Math.PI * Radius * Radius;
}
}
class Program {
static void Main(string[] args) {
// 创建三个对象
Rectangle rectangle = new Rectangle(3, 4);
Square square = new Square(5);
Circle circle = new Circle(2);
// 输出三种图形的面积
Console.WriteLine($"长方形的面积为:{rectangle.Area()}");
Console.WriteLine($"正方形的面积为:{square.Area()}");
Console.WriteLine($"圆形的面积为:{circle.Area()}");
}
}
```
输出结果为:
```
长方形的面积为:12
正方形的面积为:25
圆形的面积为:12.566370614359172
```
阅读全文
相关推荐
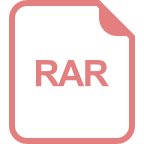
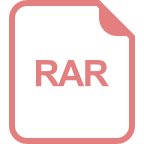


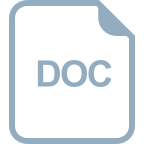
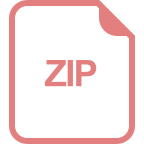







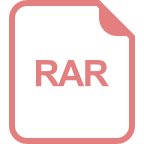
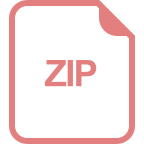
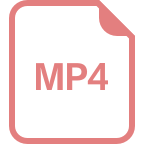