计算几种不同的三维图形的表面积和体积。这里定义一个抽象类CShape,将其成员函数显示基本图形信息函数DispAttr()、计算表面积函数SArea()和计算体积函数Volume()定义为纯虚函数。根据此抽象类派生出球体、正方体、长方体和圆柱体,并分别重新实现基类中的纯虚函数来显示输出计算结果。
时间: 2024-03-14 11:48:17 浏览: 18
好的,这是一个关于计算不同三维图形的表面积和体积的问题,并且需要使用抽象类CShape和其派生类来实现。下面是代码实现:
```c++
#include<iostream>
using namespace std;
//抽象类
class CShape {
public:
virtual void DispAttr() = 0; //显示基本图形信息函数
virtual double SArea() = 0; //计算表面积函数
virtual double Volume() = 0; //计算体积函数
};
//球体派生类
class CSphere : public CShape {
private:
double radius;
public:
CSphere(double r) { radius = r; }
void DispAttr() { cout << "球体半径为:" << radius << endl; }
double SArea() { return 4 * 3.14 * radius * radius; }
double Volume() { return 4 * 3.14 * radius * radius * radius / 3; }
};
//正方体派生类
class CCube : public CShape {
private:
double side;
public:
CCube(double s) { side = s; }
void DispAttr() { cout << "正方体边长为:" << side << endl; }
double SArea() { return 6 * side * side; }
double Volume() { return side * side * side; }
};
//长方体派生类
class CRectangle : public CShape {
private:
double length, width, height;
public:
CRectangle(double l, double w, double h) { length = l; width = w; height = h; }
void DispAttr() { cout << "长方体长为:" << length << ",宽为:" << width << ",高为:" << height << endl; }
double SArea() { return 2 * (length*width + length*height + width*height); }
double Volume() { return length * width * height; }
};
//圆柱体派生类
class CCylinder : public CShape {
private:
double radius, height;
public:
CCylinder(double r, double h) { radius = r; height = h; }
void DispAttr() { cout << "圆柱体底面半径为:" << radius << ",高为:" << height << endl; }
double SArea() { return 2 * 3.14 * radius * radius + 2 * 3.14 * radius * height; }
double Volume() { return 3.14 * radius * radius * height; }
};
int main() {
CShape* pShape; //指向抽象类的指针
//球体
pShape = new CSphere(5);
pShape->DispAttr();
cout << "球体表面积为:" << pShape->SArea() << endl;
cout << "球体体积为:" << pShape->Volume() << endl;
delete pShape;
//正方体
pShape = new CCube(10);
pShape->DispAttr();
cout << "正方体表面积为:" << pShape->SArea() << endl;
cout << "正方体体积为:" << pShape->Volume() << endl;
delete pShape;
//长方体
pShape = new CRectangle(3, 4, 5);
pShape->DispAttr();
cout << "长方体表面积为:" << pShape->SArea() << endl;
cout << "长方体体积为:" << pShape->Volume() << endl;
delete pShape;
//圆柱体
pShape = new CCylinder(2, 5);
pShape->DispAttr();
cout << "圆柱体表面积为:" << pShape->SArea() << endl;
cout << "圆柱体体积为:" << pShape->Volume() << endl;
delete pShape;
return 0;
}
```
以上程序中,通过抽象类CShape定义了计算表面积、体积和显示基本信息的纯虚函数。再通过CShape的派生类来实现这些函数。最后在主函数中,通过指向抽象类的指针来调用各个派生类的函数,实现计算不同三维图形的表面积和体积。
相关推荐
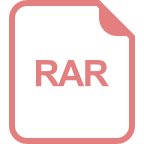
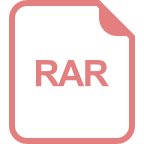










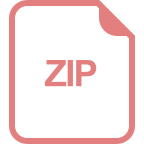
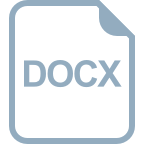