Java Swing 实现字母拼写单词小游戏的代码
时间: 2023-11-13 18:04:19 浏览: 134
这是一个简单的Java Swing实现字母拼写单词小游戏的代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class SpellingGame extends JFrame implements ActionListener {
// 单词列表
private String[] words = {"apple", "banana", "cherry", "orange", "pear"};
// 当前单词
private String currentWord;
// 当前单词的字母列表
private char[] currentLetters;
// 当前字母序号
private int currentLetterIndex;
// 游戏界面的组件
private JLabel wordLabel;
private JTextField letterTextField;
private JButton startButton;
private JButton nextButton;
public SpellingGame() {
setTitle("字母拼写单词小游戏");
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(400, 150);
setLocationRelativeTo(null);
// 布局
setLayout(new GridLayout(3, 2));
// 单词标签
wordLabel = new JLabel("", JLabel.CENTER);
add(wordLabel);
// 字母输入框
letterTextField = new JTextField(10);
letterTextField.setEnabled(false);
add(letterTextField);
// 开始按钮
startButton = new JButton("开始");
startButton.addActionListener(this);
add(startButton);
// 下一个字母按钮
nextButton = new JButton("下一个字母");
nextButton.setEnabled(false);
nextButton.addActionListener(this);
add(nextButton);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
// 开始游戏
currentWord = words[(int) (Math.random() * words.length)];
currentLetters = currentWord.toCharArray();
currentLetterIndex = 0;
wordLabel.setText(currentWord.charAt(0) + "");
letterTextField.setEnabled(true);
letterTextField.setText("");
startButton.setEnabled(false);
nextButton.setEnabled(true);
} else if (e.getSource() == nextButton) {
// 检查当前字母是否正确
char currentLetter = currentLetters[currentLetterIndex];
char inputLetter = letterTextField.getText().toLowerCase().charAt(0);
if (currentLetter == inputLetter) {
// 字母正确,显示下一个字母
currentLetterIndex++;
if (currentLetterIndex < currentLetters.length) {
wordLabel.setText(wordLabel.getText() + currentLetters[currentLetterIndex]);
letterTextField.setText("");
} else {
// 所有字母都拼写正确,显示恭喜信息
JOptionPane.showMessageDialog(this, "恭喜你,拼写正确!", "恭喜", JOptionPane.INFORMATION_MESSAGE);
startButton.setEnabled(true);
nextButton.setEnabled(false);
letterTextField.setEnabled(false);
}
} else {
// 字母错误,弹出提示框
JOptionPane.showMessageDialog(this, "字母拼写错误!", "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
public static void main(String[] args) {
new SpellingGame();
}
}
```
该代码实现了一个简单的字母拼写单词小游戏,点击开始按钮后随机选择一个单词,只显示第一个字母,然后用户逐个输入其他字母,如果输入正确,就显示下一个字母,如果输入错误,就弹出提示框。当所有字母都拼写正确时,显示恭喜信息。玩家可以选择重新开始游戏。
阅读全文
相关推荐
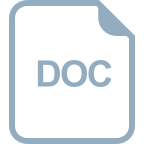
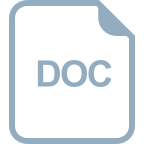
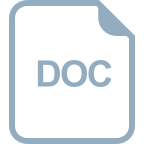
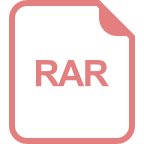
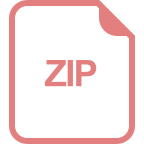
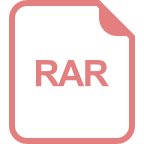
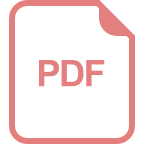
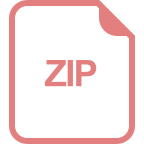
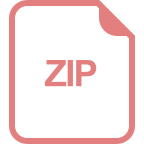
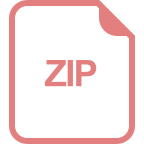
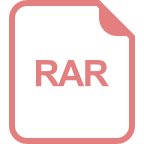