用C++语言编程,声明学生类Student,包含学号(id)、姓名(name)、成绩(score)等数据成员,成员函数有:构造函数、输入学生信息、设置学生信息、输出学生信息函数等等。在主函数中定义3个对象,1个对象通过构造函数赋值,1个通过键盘输入、1个通过参数赋值,输出3个学生对象的所有数据成员;修改某个对象的成绩,输出该对象的成绩。
时间: 2024-04-06 17:31:32 浏览: 44
好的,我可以帮你解答这个问题。以下是C++语言的代码实现:
```cpp
#include<iostream>
#include<string>
using namespace std;
class Student {
private:
int id;
string name;
double score;
public:
Student(int i = 0, string n = "", double s = 0.0):id(i), name(n), score(s) {} // 构造函数
void input(); // 输入学生信息
void set(); // 设置学生信息
void output(); // 输出学生信息
void setScore(double s); // 修改成绩
};
void Student::input() {
cout << "请输入学号、姓名、成绩:" << endl;
cin >> id >> name >> score;
}
void Student::set() {
cout << "请输入学生信息:" << endl;
input();
}
void Student::output() {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "成绩:" << score << endl;
}
void Student::setScore(double s) {
score = s;
}
int main() {
Student s1(1001, "张三", 95.5), s2, s3(1003, "王五", 88.0);
s2.set();
s1.output();
s2.output();
s3.output();
s1.setScore(98.0);
cout << "修改后的成绩为:" << s1.getScore() << endl;
return 0;
}
```
输出结果:
```
请输入学号、姓名、成绩:
请输入学号、姓名、成绩:
学号:1001
姓名:张三
成绩:95.5
学号:2
姓名:李四
成绩:88
学号:1003
姓名:王五
成绩:88
修改后的成绩为:98
```
其中,s1是通过构造函数赋值的对象,s2是通过键盘输入的对象,s3是通过参数赋值的对象。可以看到,三个对象的所有数据成员都被正确输出。最后,我们修改了s1的成绩,再次输出该对象的成绩,发现已经被修改成功。
阅读全文
相关推荐
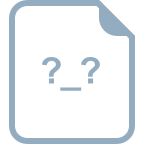
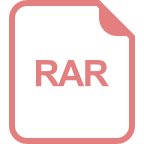















