matlab走迷宫格最短路径
时间: 2023-08-06 14:16:40 浏览: 123
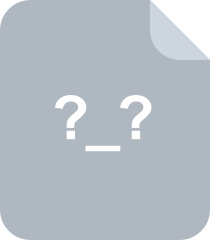
迷宫的最短路径实现

走迷宫问题可以用搜索算法来解决,其中最常见的算法是广度优先搜索和A*搜索。以下是用Matlab实现迷宫最短路径的示例代码:
```matlab
function [path, cost] = maze_solver(maze, start, goal)
% MAZE_SOLVER solves a maze using A* search algorithm
% maze: binary matrix representing the maze (0: free space, 1: obstacle)
% start: starting point (row, col) of the maze
% goal: goal point (row, col) of the maze
% path: an array of (row, col) coordinates representing the shortest path
% cost: the cost of the shortest path
% Define the heuristic function (Euclidean distance)
heuristic = @(x, y) sqrt(sum((x - y) .^ 2));
% Define the cost function (path length)
cost_function = @(x, y) norm(x - y);
% Initialize the A* search algorithm
start_node = struct('pos', start, 'g', 0, 'h', heuristic(start, goal), 'parent', []);
open_list = start_node;
closed_list = [];
while ~isempty(open_list)
% Select the node with the lowest cost in the open list
[~, idx] = min([open_list.g] + [open_list.h]);
current_node = open_list(idx);
% Check if the current node is the goal
if isequal(current_node.pos, goal)
% Reconstruct the path from start to goal
path = current_node.pos;
cost = current_node.g;
while ~isempty(current_node.parent)
path = [current_node.parent.pos; path];
current_node = current_node.parent;
end
return;
end
% Move the current node from the open list to the closed list
open_list(idx) = [];
closed_list = [closed_list, current_node];
% Generate the successors of the current node
successors = [];
for drow = -1:1
for dcol = -1:1
if abs(drow) + abs(dcol) ~= 1 % Only move in cardinal directions
continue;
end
row = current_node.pos(1) + drow;
col = current_node.pos(2) + dcol;
if row < 1 || row > size(maze, 1) || col < 1 || col > size(maze, 2) % Check bounds
continue;
end
if maze(row, col) == 1 % Check if obstacle
continue;
end
successors = [successors, struct('pos', [row, col], 'g', current_node.g + cost_function(current_node.pos, [row, col]), 'h', heuristic([row, col], goal), 'parent', current_node)];
end
end
% Add the successors to the open list if they are not already in the closed list
for i = 1:length(successors)
successor = successors(i);
if any(isequal(successor.pos, node.pos) for node = closed_list)
continue;
end
if any(isequal(successor.pos, node.pos) for node = open_list)
idx = find(arrayfun(@(x) isequal(x.pos, successor.pos), open_list));
if successor.g < open_list(idx).g % Update the cost if the new path is cheaper
open_list(idx).g = successor.g;
open_list(idx).parent = successor.parent;
end
else
open_list = [open_list, successor];
end
end
end
% If no path was found
path = [];
cost = inf;
end
```
这个函数的输入参数包括一个表示迷宫的二进制矩阵,起点和终点的坐标,输出参数是最短路径和其长度。算法使用A*搜索,其中启发函数是欧几里得距离,代价函数是路径长度。在搜索过程中,我们维护一个“开放列表”和一个“关闭列表”,用于存储待扩展的节点和已经扩展的节点。在每次迭代中,我们选择开放列表中代价最小的节点进行扩展,将其加入关闭列表,并生成其后继节点。如果后继节点不在关闭列表中,我们将其加入开放列表中,并更新其代价和父节点。最终,如果搜索成功,我们可以通过回溯父节点来重构最短路径。
阅读全文
相关推荐



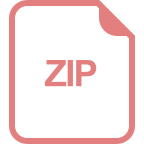
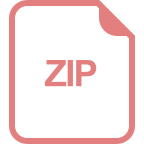
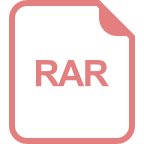
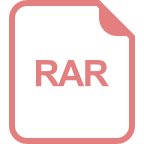
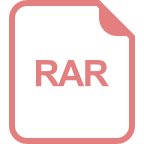
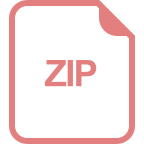
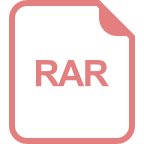
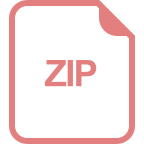



