python 关闭指定线程
时间: 2023-10-26 14:10:10 浏览: 149
在 Python 中,线程无法被直接关闭或终止。相反,可以使用 `threading.Event` 对象来通知线程退出。下面是一个简单的示例代码:
```python
import threading
class MyThread(threading.Thread):
def __init__(self, event):
threading.Thread.__init__(self)
self.stopped = event
def run(self):
while not self.stopped.wait(0.1):
# 执行线程逻辑
def stop(self):
self.stopped.set()
# 创建一个 Event 对象来控制线程的退出
stop_event = threading.Event()
# 启动线程
my_thread = MyThread(stop_event)
my_thread.start()
# 在需要时停止线程
my_thread.stop()
```
在这个例子中,`MyThread` 类继承自 `threading.Thread` 类,并接受一个 `threading.Event` 对象作为参数。线程的主要逻辑在 `run` 方法中实现,它会不断执行,直到收到 `stop_event` 对象的通知。`stop` 方法被调用时,它会将 `stop_event` 对象设置为已触发状态,从而通知线程退出。
相关问题
python 如何关闭指定线程
在Python中,如果你想要关闭一个特定的线程,通常可以利用`threading`模块中的`Thread`对象提供的一些方法来操作。但是,由于Python的全局解释器锁(GIL)的设计,不是所有的线程都可以随意停止运行,特别是那些在I/O阻塞(如网络请求、文件读写等)的状态下。
如果你想终止一个正在执行的任务(非守护线程),你可以尝试以下几种方式:
1. **设置标志**:创建一个共享变量作为线程停止的信号。当需要关闭线程时,将该变量设为True,并在线程循环内部检查这个值。如果为True,就退出循环。
```python
import threading
class MyThread(threading.Thread):
def __init__(self, flag):
super().__init__()
self._flag = flag
def run(self):
while not self._flag.is_set():
# 执行任务...
if self._flag.wait(timeout=0): # 检查标志并等待一段时间
break # 如果标志已经设置,立即退出
# 使用示例
stop_flag = threading.Event() # 创建事件对象作为信号
my_thread = MyThread(stop_flag)
my_thread.start()
# 当需要关闭线程时
stop_flag.set()
```
2. **守护线程**:如果线程是守护线程(`daemon=True`),那么主线程结束时,所有守护线程也会自动结束,不需要显式关闭。
3. **强制中断**:对于一些特殊的场景,比如使用`multiprocessing`库下的进程池,可以使用`Pool.terminate()`或`Pool.close()`来终止整个进程池,但这可能会导致未处理的数据丢失。
然而,需要注意的是,以上方式并不是绝对安全的,因为Python GIL的存在,它们并不能保证线程会立即停止,尤其是IO密集型的任务。此外,强行停止线程可能导致资源泄露或其他异常情况。因此,在设计多线程程序时,应尽量避免不必要的线程开启,或者选择更适合的并发模型。
Python 如何关闭线程池中 的指定线程
在Python中,如果你使用了`concurrent.futures`模块创建了一个线程池,并想关闭其中的特定线程,通常的做法是让线程完成当前任务后自然退出,因为线程池本身不会提供直接关闭单个线程的功能。线程池管理的是线程的工作任务,而不是它们的生命周期。
如果你想停止某个正在运行的任务,你可以使用`ThreadPoolExecutor.shutdown()`方法,它会等待所有已提交但还未开始执行的任务结束,然后终止剩余的空闲线程。如果你想立即停止并取消所有未完成的任务,可以使用`ThreadPoolExecutor.shutdown(wait=False)`,这将中断任务的执行。
例如:
```python
import concurrent.futures
# 创建线程池
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
# 提交任务
future = executor.submit(long_running_task, argument)
# 想关闭某项任务,需要等它进入工作队列
if some_condition:
future.cancel() # 取消任务
print(f"Task cancelled: {future.cancelled()}")
# 使用shutdown方法关闭整个线程池
executor.shutdown()
```
需要注意的是,只有当线程可以安全地被打断(即不是阻塞状态),`cancel()`方法才会成功。如果任务已经执行到某个不可中断的状态(如系统调用),那么取消操作将无效。
阅读全文
相关推荐
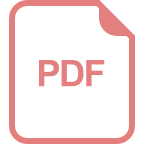
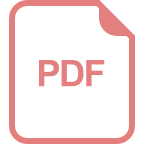
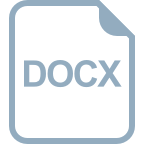
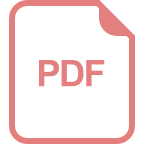
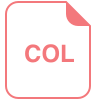



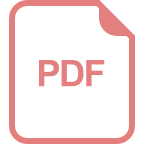
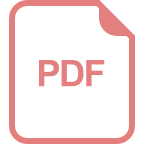
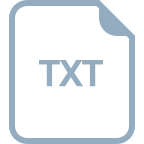
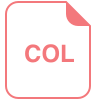
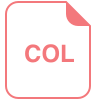
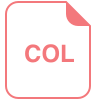
