delphi a*寻路
时间: 2023-07-23 12:38:31 浏览: 142
Delphi是一种编程语言,可以使用它来实现A*寻路算法。下面是一些实现A*寻路算法的基本步骤:
1. 创建一个二维数组来表示地图。
2. 使用一个结构体来表示每一个格子,包含以下信息:坐标、是否可通过、该点到起点的距离、该点到终点的预估距离(使用曼哈顿距离或欧几里得距离等)和该点是否已经被访问过。
3. 创建一个优先队列,用于存储待访问的格子。
4. 将起点加入优先队列,并将起点到起点的距离设为0。
5. 每次从优先队列中取出距离起点最短的格子,对其周围的8个格子进行访问。如果该格子未被访问过,并且可通过,就更新该格子到起点的距离和到终点的预估距离,并将其加入优先队列中。
6. 重复步骤5,直到找到终点或优先队列为空。
下面是一个简单的Delphi实现:
```delphi
type
TPoint = record
x, y: integer;
end;
TNode = record
pos: TPoint;
passable, visited: boolean;
g, h: integer;
parent: ^TNode;
end;
var
map: array of array of TNode;
openList: TQueue<TNode>;
function ManhattanDistance(p1, p2: TPoint): integer;
begin
Result := Abs(p1.x - p2.x) + Abs(p1.y - p2.y);
end;
function AStar(start, goal: TPoint): TList<TPoint>;
var
currentNode, neighbor: ^TNode;
i, j, newG: integer;
begin
// 初始化地图
SetLength(map, MAP_WIDTH, MAP_HEIGHT);
for i := 0 to MAP_WIDTH - 1 do
for j := 0 to MAP_HEIGHT - 1 do
begin
map[i][j].pos.x := i;
map[i][j].pos.y := j;
map[i][j].passable := IsPassable(i, j); // 根据实际情况实现该函数
map[i][j].visited := false;
map[i][j].g := MaxInt;
end;
// 初始化起点
currentNode := @map[start.x][start.y];
currentNode^.g := 0;
currentNode^.h := ManhattanDistance(currentNode^.pos, goal);
currentNode^.parent := nil;
openList.Enqueue(currentNode^);
// A*搜索
while not openList.IsEmpty do
begin
currentNode := @openList.Dequeue;
if (currentNode^.pos.x = goal.x) and (currentNode^.pos.y = goal.y) then
begin
// 找到了终点,回溯路径
Result := TList<TPoint>.Create;
while currentNode <> nil do
begin
Result.Add(currentNode^.pos);
currentNode := currentNode^.parent;
end;
Exit;
end;
currentNode^.visited := true;
for i := -1 to 1 do
for j := -1 to 1 do
begin
if (i = 0) and (j = 0) then
continue;
if (currentNode^.pos.x + i < 0) or (currentNode^.pos.x + i >= MAP_WIDTH) or
(currentNode^.pos.y + j < 0) or (currentNode^.pos.y + j >= MAP_HEIGHT) then
continue;
neighbor := @map[currentNode^.pos.x + i][currentNode^.pos.y + j];
if not neighbor^.passable or neighbor^.visited then
continue;
newG := currentNode^.g + 1; // 假设每个格子的代价都为1
if newG < neighbor^.g then
begin
neighbor^.g := newG;
neighbor^.h := ManhattanDistance(neighbor^.pos, goal);
neighbor^.parent := currentNode;
openList.Enqueue(neighbor^);
end;
end;
end;
// 没有找到路径
Result := nil;
end;
```
需要注意的是,上面的代码中使用了Delphi自带的泛型队列TQueue和泛型列表TList。如果你使用的是较老的Delphi版本,可能需要手动实现这些数据结构。另外,该代码中还使用了一个IsPassable函数来判断一个格子是否可通过,你需要根据实际情况实现该函数。
阅读全文
相关推荐
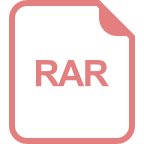
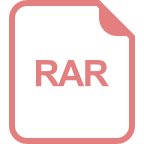
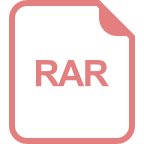
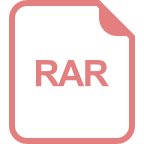

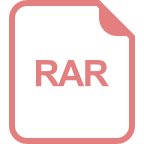
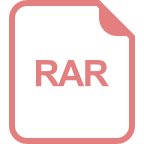
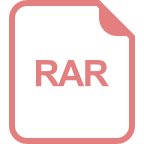
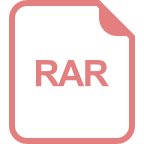
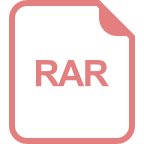
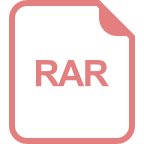
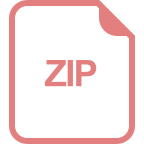
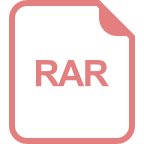
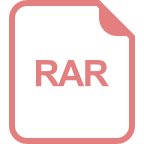
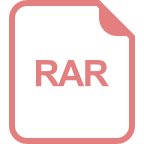
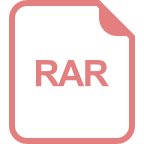