用python设计我的英文字典,所有的单词组成一个列表,每个单词与注释成为一个字典,程序的功能就是管理这样一组单词记录,程序有查找单词(用二分查找法在words中查找单词w)、增加单词、更新注释、删除单词、显示单词等功能
时间: 2024-05-06 14:16:43 浏览: 14
好的,下面是一个简单的实现:
```python
words = [{'word': 'apple', 'definition': 'a round fruit with a red or green skin and firm white flesh'},
{'word': 'banana', 'definition': 'a long curved fruit which grows in clusters and has soft pulpy flesh and yellow skin when ripe'},
{'word': 'cherry', 'definition': 'a small, round fruit with a red or black skin and a hard stone'},
{'word': 'dog', 'definition': 'a domesticated carnivorous mammal with a pointed snout and furry coat'}]
def binary_search(word, words):
low = 0
high = len(words) - 1
while low <= high:
mid = (low + high) // 2
if words[mid]['word'] == word:
return mid
elif words[mid]['word'] < word:
low = mid + 1
else:
high = mid - 1
return None
def find_word(word, words):
idx = binary_search(word, words)
if idx is not None:
print(words[idx]['word'], ':', words[idx]['definition'])
else:
print('Word not found')
def add_word(word, definition, words):
idx = binary_search(word, words)
if idx is not None:
print('Word already exists')
else:
words.append({'word': word, 'definition': definition})
words.sort(key=lambda x: x['word'])
print('Word added successfully')
def update_definition(word, definition, words):
idx = binary_search(word, words)
if idx is not None:
words[idx]['definition'] = definition
print('Definition updated successfully')
else:
print('Word not found')
def delete_word(word, words):
idx = binary_search(word, words)
if idx is not None:
del words[idx]
print('Word deleted successfully')
else:
print('Word not found')
def display_words(words):
for word in words:
print(word['word'], ':', word['definition'])
# 测试代码
find_word('apple', words)
add_word('cat', 'a small carnivorous mammal with soft fur, a short snout, and retractable claws', words)
update_definition('dog', 'a domesticated carnivorous mammal with a pointed snout and fur', words)
delete_word('cherry', words)
display_words(words)
```
这个程序使用了一个列表 `words` 来存储所有的单词和注释,每个单词和注释都被存储为一个字典。主要的功能函数包括:
- `binary_search(word, words)`:用二分查找法在 `words` 中查找单词 `word`,返回单词在列表中的下标,如果找不到则返回 `None`。
- `find_word(word, words)`:查找单词并显示其注释。
- `add_word(word, definition, words)`:添加一个新单词和注释。
- `update_definition(word, definition, words)`:更新一个已有单词的注释。
- `delete_word(word, words)`:删除一个已有单词。
- `display_words(words)`:显示所有单词和注释。
你可以根据需要修改这些函数来满足自己的需求。
相关推荐
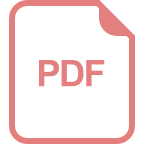
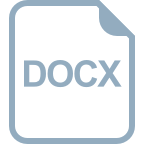















