import string import random x = int(input()) n = int(input()) m = int(input()) random.seed(x) chars = string.ascii_lowercase + string.ascii_uppercase + string.digits for i in range(n): password = ''.join(random.choice(chars) for _ in range(m)) print(password)
时间: 2024-02-24 20:57:06 浏览: 155
这段代码中,首先通过 `import` 语句引入了 `string` 和 `random` 两个模块。然后通过 `input()` 函数获取了三个整数值 `x`、`n` 和 `m`,分别表示随机数种子、生成密码的个数和每个密码的长度。
接下来,通过 `random.seed(x)` 函数设置了随机数种子,保证每次生成的随机数序列相同。然后将所有的小写字母、大写字母和数字字符合并到一个字符串 `chars` 中。
最后,通过 `for` 循环生成 `n` 个随机密码,每个密码的长度为 `m`。具体地,使用 `random.choice()` 函数从 `chars` 中随机选择 `m` 个字符,并使用 `join()` 函数将它们连接成一个字符串。最后将生成的密码打印出来。
需要注意的是,该段代码的缩进存在问题,需要进行调整,否则会导致语法错误。正确的代码如下:
```python
import string
import random
x = int(input())
n = int(input())
m = int(input())
random.seed(x)
chars = string.ascii_lowercase + string.ascii_uppercase + string.digits
for i in range(n):
password = ''.join(random.choice(chars) for _ in range(m))
print(password)
```
相关问题
解释这段代码package question7; import java.io.DataOutputStream; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.PrintWriter; import java.util.ArrayList; import java.util.HashSet; import java.util.Scanner; import java.util.Set; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.Random; public class Test { public static void main(String args[]){ Test test=new Test(); Scanner input=new Scanner(System.in); String s1=new String(); s1=input.nextLine(); String[] s2 = s1.split(" "); int[] l=new int[s2.length]; for(int i=0 ; i<s2.length;i++){ l[i]=Integer.parseInt(s2[i]); } test.write("test.txt", l); int[] readlist=test.read("test.txt",l); isPrime isprime=new isPrime(readlist); for(int i=1;i<=10;i++){ new Thread(isprime).start(); } try { Thread.sleep(1000); //1000 毫秒,也就是1秒. } catch(InterruptedException ex) { Thread.currentThread().interrupt(); } Set<Integer> set=new HashSet(); set=isprime.getSet(); System.out.println("素数set"+set); System.out.println("输入查询数字"); int num=input.nextInt(); if(set.contains(num)){ System.out.println(num+"是素数"); } else System.out.println(num+"不是是素数"); } public void write(String filename,int a[]){ File file = new File(filename); try { PrintWriter output=new PrintWriter(file); for(int i=0;i<a.length;i++){ output.print(a[i]+" "); } output.close(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public int[] read(String filename,int a[]){ File file=new File(filename); try { Scanner input=new Scanner(file); int i=0; while(input.hasNext()){ a[i]=input.nextInt(); i++; } } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } return a; } }
这段代码是一个 Java 程序,主要功能是读取用户输入的一串整数,将其写入文件 test.txt,然后又从这个文件中读取整数列表,并查找出其中的素数并存储在一个 HashSet 中,最后判断用户输入的数字是否为素数。具体实现包括定义了一个 isPrime 类用于判断一个数字是否为素数,使用了多线程来处理多个数字的判断,使用了 HashSet 来存储素数列表。
对于package 课程设计; import java.util.Scanner; public class suanshuyunsuan { public static void main(String[] args) { int yourGuess; int m[]=new int [10]; int sum=0; for(int l=0;l<5;l++) { int a=(int)(Math.round(1+99*Math.random())); int b=(int)(Math.round(1+99*Math.random())); m[l]=a-b; System.out.println(a+"-"+b+"="+" "); System.out.println("yourGuess:"); Scanner input=new Scanner(System.in); yourGuess=input.nextInt(); if(yourGuess==m[l]) { System.out.println("对"); sum=sum+10; } else System.out.println("错"); } for(int l=5;l<10;l++) { int a=(int)(Math.round(1+99*Math.random())); int b=(int)(Math.round(1+99*Math.random())); m[l]=a+b; System.out.println(a+"+"+b+"="+" "); System.out.println("yourGuess:"); Scanner input=new Scanner(System.in); yourGuess=input.nextInt(); if(yourGuess==m[l]) { System.out.println("对"); sum=sum+10; } else System.out.println("错"); } System.out.println("最终得分="+sum); } }的课程设计怎么写
这段代码是一个简单的Java程序,用于生成10道100以内的加减法题目,并要求用户输入答案进行判断,并计算最终得分。下面是对这段代码的课程设计的分析和改进建议:
1. 首先,在课程设计的引言部分,可以简要介绍这段代码的功能和目的,即实现生成加减法题目、用户答题和得分计算的功能。
2. 在需求分析部分,可以详细描述这段代码的功能需求和性能需求,例如生成题目的范围、用户答题的方式等。
3. 在系统设计部分,可以对代码进行结构化和模块化的改进。可以将生成题目和判断答案的逻辑封装成独立的方法,提高代码的可读性和可维护性。
4. 在系统实现部分,可以给出改进后的代码实现,并提供关键代码片段,并解释其功能和实现原理。
5. 在系统测试与结果分析部分,可以描述测试方法和测试数据集的选择,并对程序进行功能测试和边界测试,并记录测试结果。同时,对测试结果进行分析,包括系统的准确性和效率等方面。
6. 结果与讨论部分,可以对系统的实现进行评估,并提出改进和优化的建议。可以讨论代码的可扩展性、可维护性以及用户体验等方面的问题。
7. 总结与展望部分,总结课程设计的完成情况和达到的目标,并回顾设计过程中的经验和教训,并展望未来对该系统的改进和发展方向。
8. 参考文献部分,引用使用到的参考文献,包括教材、论文、网站等。
9. 附录部分,提供完整的改进后的代码清单和运行结果示例。
以上是对这段代码进行课程设计的一般步骤和结构,您可以根据具体情况进行调整和扩展。在论文中,重点描述这段代码的实现思路和功能,以及对代码的改进和优化。希望以上内容对您的课程设计有所帮助!祝您顺利完成课程设计!
阅读全文
相关推荐


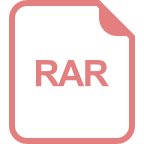














<%-- 作者:GGG166 --%> <%@page contentType="text/html;charset=gb2312" %> <%@page import="java.util.*"%> <html> <body> <%! Vector v=new Vector();//动态数组 int i=0; ServletContext application; synchronized void leaveWord(String s){//方法声明,用于在添加评论 application=getServletContext(); i++; v.add("No"+i+","+s); application.setAttribute("Mess",v); } %> <% request.setCharacterEncoding("gb2312");//乱码处理 String name=request.getParameter("name");//接收姓名 String title=request.getParameter("title");//接收标题 String message=request.getParameter("message");//接收评论 if(name==null){ name="guest"+(int)(Math.random()*10000); } if(title==null){ title="无标题"; } if(message==null){ message="无信息"; } String s=name+"#"+title+"#"+message; leaveWord(s); out.print("你的评论已提交!"); %> 返回留言页面 </body> </html>
