使用react实现类似Antd的对话框Modal组件。只需要实现基本功能,不需要像Antd那样功能完善 对话框组件有标题、内容区和底部,并且底部有两个按钮ok和cancel Modal组件要求支持title(标题),visible(对话框是否可见) onOk(点击按钮的回调函数)和onCancel(点击取消按钮的回调函数)属性Prop
时间: 2024-01-24 17:17:14 浏览: 20
好的,下面是一个基本的Modal组件的实现示例:
```javascript
import React from 'react';
import ReactDOM from 'react-dom';
class Modal extends React.Component {
constructor(props) {
super(props);
this.state = {
visible: this.props.visible || false,
};
}
handleOk = () => {
if (this.props.onOk) {
this.props.onOk();
}
this.setState({ visible: false });
};
handleCancel = () => {
if (this.props.onCancel) {
this.props.onCancel();
}
this.setState({ visible: false });
};
render() {
const { title, children } = this.props;
const { visible } = this.state;
return visible ? (
<div className="modal-wrapper">
<div className="modal">
<div className="modal-header">
<h4>{title}</h4>
</div>
<div className="modal-body">{children}</div>
<div className="modal-footer">
<button onClick={this.handleCancel}>Cancel</button>
<button onClick={this.handleOk}>Ok</button>
</div>
</div>
</div>
) : null;
}
}
const App = () => {
const handleOk = () => {
console.log('Ok clicked');
};
const handleCancel = () => {
console.log('Cancel clicked');
};
return (
<div>
<button onClick={() => ReactDOM.render(<Modal visible />, document.getElementById('root'))}>
Show Modal
</button>
<Modal title="Modal Title" visible={false} onOk={handleOk} onCancel={handleCancel}>
<p>This is the modal content.</p>
</Modal>
</div>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
```
在这个示例中,我们定义了一个Modal组件,它有三个props:title、visible和children。title是标题,visible表示对话框是否可见,children是内容区域的内容。我们在组件的constructor中初始化了state,将visible的初始值设置为props中传入的visible值或false。当用户点击ok或cancel按钮时,会调用对应的回调函数,并将visible设置为false,关闭对话框。
在Modal组件的render方法中,我们根据visible的值来控制Modal组件的显示。当visible为true时,渲染Modal的内容,包括标题、内容区域和底部的按钮。当visible为false时,不渲染任何内容。我们还定义了一个App组件来展示Modal组件的用法。在App组件中,我们定义了handleOk和handleCancel两个回调函数,并在render方法中渲染了一个按钮,用来打开Modal组件。我们也渲染了一个初始状态为不可见的Modal组件,并将handleOk和handleCancel作为props传递给Modal组件。
以上就是一个简单的Modal组件的实现示例,你可以根据需要进行修改和完善。
相关推荐
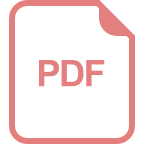
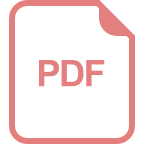
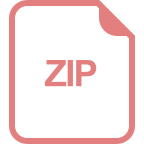














