解释一段python代码 class KalmanFilter(object): def init(self, dim_x, dim_z, dim_u=0): if dim_x < 1: raise ValueError('dim_x must be 1 or greater') if dim_z < 1: raise ValueError('dim_z must be 1 or greater') if dim_u < 0: raise ValueError('dim_u must be 0 or greater') self.dim_x = dim_x self.dim_z = dim_z self.dim_u = dim_u self.x = zeros((dim_x, 1)) # state self.P = eye(dim_x) # uncertainty covariance self.Q = eye(dim_x) # process uncertainty self.B = None # control transition matrix self.F = eye(dim_x) # state transition matrix self.H = zeros((dim_z, dim_x)) # Measurement function self.R = eye(dim_z) # state uncertainty self._alpha_sq = 1. # fading memory control self.M = np.zeros((dim_z, dim_z)) # process-measurement cross correlation self.z = np.array([[None]*self.dim_z]).T # gain and residual are computed during the innovation step. We # save them so that in case you want to inspect them for various # purposes self.K = np.zeros((dim_x, dim_z)) # kalman gain self.y = zeros((dim_z, 1)) self.S = np.zeros((dim_z, dim_z)) # system uncertainty self.SI = np.zeros((dim_z, dim_z)) # inverse system uncertainty # identity matrix. Do not alter this. self._I = np.eye(dim_x) # these will always be a copy of x,P after predict() is called self.x_prior = self.x.copy() self.P_prior = self.P.copy() # these will always be a copy of x,P after update() is called self.x_post = self.x.copy() self.P_post = self.P.copy() # Only computed only if requested via property self._log_likelihood = log(sys.float_info.min) self._likelihood = sys.float_info.min self._mahalanobis = None self.inv = np.linalg.inv
时间: 2024-02-14 12:35:40 浏览: 22
这段Python代码是KalmanFilter类的初始化方法。在这个方法中,首先会检查dim_x、dim_z和dim_u是否符合要求,如果不符合就会抛出ValueError异常。然后会根据参数的值初始化KalmanFilter对象的各个属性,包括状态量的维度dim_x、观测量的维度dim_z、控制量的维度dim_u。初始化时,状态量x被初始化为一个dim_x行1列的零向量,不确定性协方差P被初始化为dim_x阶单位矩阵,过程不确定性Q也被初始化为dim_x阶单位矩阵。控制转移矩阵B、状态转移矩阵F、测量函数H、状态不确定性R、过程-测量交叉相关M、增长记忆控制参数_alpha_sq、测量残差z、卡尔曼增益K、残差y、系统不确定性S和其逆矩阵SI等都被初始化为相应的大小的零矩阵或数组。这个类还包含一个求逆矩阵的方法inv。
相关问题
解释一段python代码 class KalmanFilter(object): def init(self, dim_x, dim_z, dim_u=0): if dim_x < 1: raise ValueError('dim_x must be 1 or greater') if dim_z < 1: raise ValueError('dim_z must be 1 or greater')
这段Python代码定义了一个名为KalmanFilter的类,并定义了一个名为init的方法。该方法接受三个参数:dim_x,dim_z和dim_u,其中dim_x和dim_z是必需的,而dim_u是可选的,默认值为0。
在方法的第一行,通过if语句检查dim_x是否小于1,如果是,则会引发一个ValueError异常,提示dim_x必须大于或等于1。同样的操作也会检查dim_z是否小于1,如果是,则会引发相同的异常。
这段代码的功能是检查传入的参数是否合法,如果不合法则抛出异常,以保证代码的健壮性和可靠性。
翻译一段python代码 import torch class KalmanFilter: def __init__(self, F, H, Q, R, x0, P0): self.F = F self.H = H self.Q = Q self.R = R self.x = x0 self.P = P0 def predict(self): self.x = torch.matmul(self.F, self.x) self.P = torch.matmul(torch.matmul(self.F, self.P), torch.transpose(self.F, 0, 1)) + self.Q def update(self, z): y = z - torch.matmul(self.H, self.x) S = torch.matmul(torch.matmul(self.H, self.P), torch.transpose(self.H, 0, 1)) + self.R K = torch.matmul(torch.matmul(self.P, torch.transpose(self.H, 0, 1)), torch.inverse(S)) self.x = self.x + torch.matmul(K, y) self.P = torch.matmul(torch.eye(self.F.shape[0]) - torch.matmul(K, self.H), self.P)
这段 Python 代码定义了一个卡尔曼滤波器(KalmanFilter)类,包含了初始化函数 __init__,以及预测函数 predict 和更新函数 update。
在 __init__ 函数中,传入了 F、H、Q、R、x0 和 P0 作为参数,分别表示状态转移矩阵、观测矩阵、过程噪声协方差矩阵、观测噪声协方差矩阵、初始状态向量和初始协方差矩阵。这些参数被分别赋值给类的属性 F、H、Q、R、x 和 P。
在 predict 函数中,根据卡尔曼滤波器的预测步骤,通过矩阵乘法更新状态向量和协方差矩阵。
在 update 函数中,根据卡尔曼滤波器的更新步骤,计算卡尔曼增益 K,通过矩阵乘法更新状态向量和协方差矩阵。其中,z 表示观测向量,y 表示观测向量与预测值之间的差值,S 表示观测噪声协方差矩阵和协方差矩阵的加权和。
相关推荐
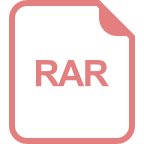
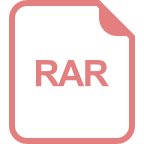
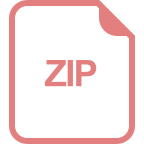














