yolov8检测单张图片
时间: 2024-01-14 08:21:46 浏览: 254
以下是使用YOLOv8检测单张图片的步骤:
1. 导入所需的库和模型:
```python
import cv2
import numpy as np
from yolov8 import YOLOv8
# 加载YOLOv8模型
model = YOLOv8()
```
2. 加载预训练权重:
```python
model.load_weights('yolov8.weights')
```
3. 定义类别标签:
```python
class_labels = ['person', 'car', 'bicycle', ...] # 根据实际情况修改类别标签
```
4. 加载待检测的图片:
```python
image = cv2.imread('image.jpg') # 根据实际情况修改图片路径
```
5. 图片预处理:
```python
input_image = model.preprocess_input(image)
```
6. 进行目标检测:
```python
detections = model.detect_objects(input_image)
```
7. 解析检测结果:
```python
for detection in detections:
class_index = detection['class_index']
class_label = class_labels[class_index]
confidence = detection['confidence']
x, y, w, h = detection['bbox']
# 在图片上绘制检测框和类别标签
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, f'{class_label}: {confidence:.2f}', (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
```
8. 显示检测结果:
```python
cv2.imshow('Detection Result', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
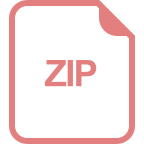
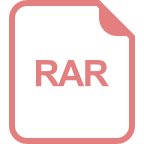
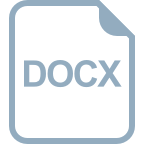





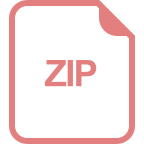
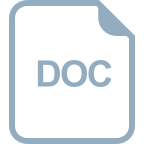
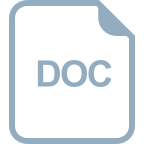
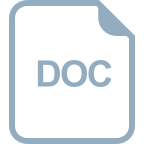
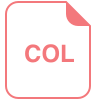





