import React, { useState } from 'react' import calendar from 'js-calendar-converter' const j = ['立春', '惊蛰', '清明', '立夏', '芒种', '小暑', '立秋', '白露', '寒露', '立冬', '大雪', '小寒'] const News = () => { const formatDate = (date) => { const year = date.getFullYear() const month = date.getMonth() + 1 const day = date.getDate() const hour = date.getHours() const minute = date.getMinutes() const lunarDate = calendar.solar2lunar(year, month, day) console.log(lunarDate) return `${year}年${month}月${day}日 ${hour}时${minute}分` } const handleConfirm = (val) => { formatDate(val) } return ( <div> <DatePicker onConfirm={handleConfirm} /> </div> ) } 以上代码里,变量j里的内容为我国十二节气的名称。 打印lunarDate结果显示有很多参数,包括参数Term代表某一节气的名称,参数cYear代表当前选中的阳历年,参数cMonth代表当前选中的阳历月,cDay代表当前选中的阳历日,参数date代表当前选中的阳历年月日。 使用react、es6语法和Javascript修改以上代码,实现获取每一年每一个十二节气的阳历日期。
时间: 2023-12-29 12:03:34 浏览: 74
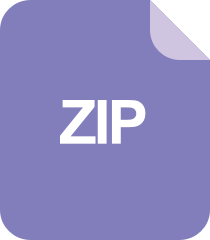
simple-react-calendar:基于React的简单日历组件,用于选择日期和日期范围
可以使用循环遍历的方式获取每一年的十二节气阳历日期。具体实现如下:
```
import React, { useState } from 'react'
import calendar from 'js-calendar-converter'
const j = ['立春', '惊蛰', '清明', '立夏', '芒种', '小暑', '立秋', '白露', '寒露', '立冬', '大雪', '小寒']
const News = () => {
const [solarDates, setSolarDates] = useState([])
const getSolarDates = (year) => {
const solarDatesOfYear = []
for (let i = 0; i < j.length; i++) {
const solarDate = calendar.lunar2solar(year, i * 2 + 3, 1) // 通过农历日期计算阳历日期
solarDatesOfYear.push({
term: j[i],
year: solarDate.cYear,
month: solarDate.cMonth,
day: solarDate.cDay,
date: `${solarDate.cYear}-${solarDate.cMonth}-${solarDate.cDay}`
})
}
return solarDatesOfYear
}
const handleConfirm = (val) => {
const year = val.getFullYear()
const solarDatesOfYear = getSolarDates(year)
setSolarDates(solarDatesOfYear)
}
return (
<div>
<DatePicker
onConfirm={handleConfirm}
/>
<ul>
{solarDates.map((solarDate, index) => (
<li key={index}>{solarDate.term}: {solarDate.date}</li>
))}
</ul>
</div>
)
}
```
首先,在组件中添加一个状态变量`solarDates`,用于存储每一年的十二节气阳历日期。
在`getSolarDates`函数中,使用循环遍历的方式获取每一年的十二节气阳历日期。具体实现为,通过计算每个节气对应的农历日期,然后将其转换为阳历日期。最后将阳历日期存储到`solarDatesOfYear`数组中。
在`handleConfirm`函数中,获取选中的年份,然后调用`getSolarDates`函数获取该年份的十二节气阳历日期,并将其存储到`solarDates`状态变量中。
在组件中渲染`solarDates`数组中的阳历日期。
阅读全文
相关推荐
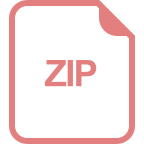
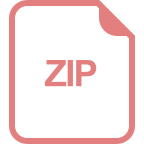


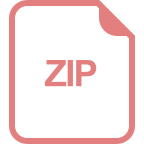

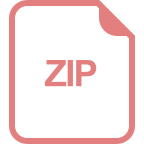
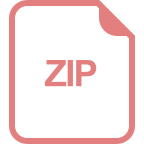
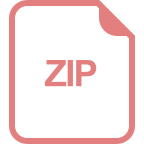
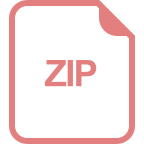
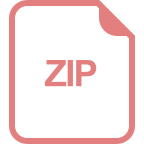
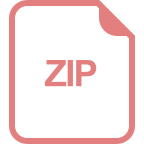
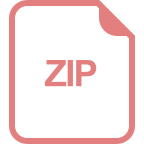
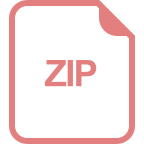
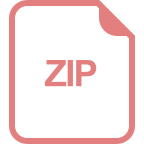
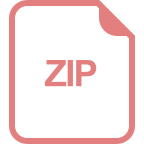
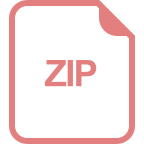
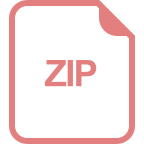