写一段代码python,对图像进行gamma correction
时间: 2024-02-02 13:02:49 浏览: 184
Sure, here's some Python code for gamma correction of an image:
```python
import cv2
# Load the image
img = cv2.imread('image.jpg')
# Define the gamma value
gamma = 1.5
# Apply the gamma correction
gamma_correction = img ** (1/gamma)
# Save the output image
cv2.imwrite('gamma_corrected_image.jpg', gamma_correction)
```
In this code, we load an image using OpenCV, define the gamma value (1.5 in this example), and apply the gamma correction using the power operator. Finally, the corrected image is saved to disk.
相关问题
数字图像处理中用python对素材图像进行gamma变换
在数字图像处理中,伽马变换(Gamma Correction)是一种常见的色彩校正技术,用于调整图像的整体亮度和对比度,尤其是在视频和计算机显示器上,因为它们通常使用非线性的灰度到颜色转换。Python提供了很多库来进行这种操作,比如PIL(Python Imaging Library)和OpenCV。
以下是使用Python和PIL库进行伽马变换的基本步骤:
1. **导入所需库**:
```python
from PIL import Image
import math
```
2. **读取图像**:
```python
img = Image.open('your_image.jpg')
```
3. **获取像素值并应用伽马变换**:
伽马变换公式通常是 `I_out = I_in^(1/gamma)`,其中 `I_in` 是输入像素值,`I_out` 是输出像素值,`gamma` 是伽马系数(通常取值为0.45至2.2之间)。这里我们假设输入图像的每个像素是一个0-255范围内的整数。
```python
def apply_gamma_correction(pixel, gamma):
return int((pixel / 255) ** (1 / gamma) * 255)
gamma = 2.2 # 可以根据需要选择合适的伽马值
new_pixels = [[apply_gamma_correction(p, gamma) for p in row] for row in img.getdata()]
```
4. **创建新的Image对象并保存结果**:
```python
new_img = Image.frombytes(img.mode, img.size, bytes(new_pixels))
new_img.save('gamma_corrected_image.jpg')
```
帮我写一个rgb gamma的python代码
当然,下面是一个简单的RGB Gamma校正的Python代码示例:
```python
import numpy as np
def gamma_correction(image, gamma):
# 对每个通道进行Gamma校正
corrected_image = np.power(image, gamma)
# 将校正后的像素值缩放到0-255范围
corrected_image = (corrected_image * 255).astype(np.uint8)
return corrected_image
# 示例用法
# 创建一个3x3x3的RGB图像
image = np.array([[[255, 0, 0], [0, 255, 0], [0, 0, 255]],
[[255, 255, 0], [255, 0, 255], [0, 255, 255]],
[[255, 255, 255], [0, 0, 0], [128, 128, 128]]], dtype=np.uint8)
# 设置Gamma值(大于1增加亮度,小于1减小亮度)
gamma = 2.2
# 进行Gamma校正
corrected_image = gamma_correction(image / 255.0, gamma)
print("原始图像:")
print(image)
print("\nGamma校正后的图像:")
print(corrected_image)
```
这段代码定义了一个 `gamma_correction` 函数,它接受一个RGB图像和一个Gamma值作为参数,并返回经过Gamma校正后的图像。在示例用法中,我们创建了一个3x3x3的RGB图像,并将Gamma值设置为2.2进行校正。最后,我们打印出原始图像和校正后的图像。请注意,为了在0-255范围内显示图像,我们将像素值缩放到0-1之间进行计算,然后再缩放回0-255范围。
阅读全文
相关推荐
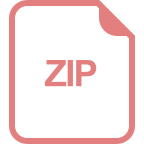
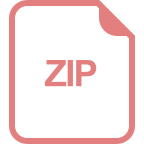
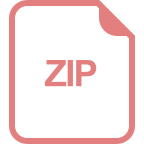













