通过vsphere golang webservice sdk 获取vmware主机、虚拟机的监控数据【一】
时间: 2023-11-24 22:26:23 浏览: 36
可以使用 vSphere SDK for Go 来获取 VMware 主机和虚拟机的监控数据。下面是一个简单的示例代码,可以获取 CPU 和内存使用率的指标数据:
```go
package main
import (
"context"
"fmt"
"net/url"
"time"
"github.com/vmware/govmomi/vim25"
"github.com/vmware/govmomi/vim25/soap"
"github.com/vmware/govmomi/vim25/types"
)
func main() {
// 连接 vSphere
u, err := soap.ParseURL("https://<vcenter>/sdk")
if err != nil {
fmt.Println(err)
return
}
u.User = url.UserPassword("<username>", "<password>")
ctx, cancel := context.WithCancel(context.Background())
defer cancel()
c, err := vim25.NewClient(ctx, u, true)
if err != nil {
fmt.Println(err)
return
}
// 获取主机和虚拟机的监控数据
perfManager := c.PerfManager()
perfQuerySpec := types.PerfQuerySpec{
Entity: types.ManagedObjectReference{
Type: "HostSystem",
Value: "<host-moid>",
},
MetricId: []types.PerfMetricId{
{
CounterId: 6, // CPU usage
Instance: "*",
},
{
CounterId: 24, // Memory usage
Instance: "*",
},
},
IntervalId: 20, // 20 seconds
Format: "normal",
}
perfQuerySpecs := []types.PerfQuerySpec{perfQuerySpec}
perfQuerySpecsResult, err := perfManager.QueryPerf(ctx, perfQuerySpecs)
if err != nil {
fmt.Println(err)
return
}
for _, perfQuerySpecResult := range perfQuerySpecsResult {
entity := perfQuerySpecResult.Entity
for _, basePerfEntityMetric := range perfQuerySpecResult.Value {
perfEntityMetric := basePerfEntityMetric.(*types.PerfEntityMetric)
instance := perfEntityMetric.Instance[0].Value
cpuUsage := perfEntityMetric.Value[0].Value[0]
memoryUsage := perfEntityMetric.Value[1].Value[0]
fmt.Printf("Entity: %s, Instance: %s, CPU usage: %f%%, Memory usage: %f%%\n", entity.Value, instance, cpuUsage, memoryUsage)
}
}
// 关闭连接
c.Logout(ctx)
}
```
在上面的代码中,需要替换 `<vcenter>`、`<username>`、`<password>` 和 `<host-moid>` 分别为 vCenter Server 的地址、用户名、密码和主机的 Managed Object ID。你还可以修改 `perfQuerySpec.MetricId` 来获取其他指标数据。
相关推荐
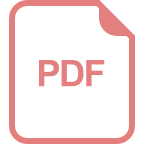
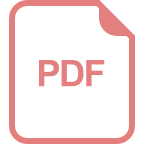














