使用二分查找法查找3,5,10,12,14,18,20,21,23,27中的20,c++完成
时间: 2024-09-20 14:16:20 浏览: 29
二分查找法(Binary Search),也叫折半查找,是一种在有序数组中寻找特定元素的搜索算法。对于给定的有序序列如3, 5, 10, 12, 14, 18, 20, 21, 23, 27,要在其中查找数字20,可以按照以下步骤使用C++编写代码:
```cpp
#include <iostream>
// 定义二分查找函数
int binarySearch(std::vector<int>& arr, int target) {
int left = 0; // 左边界
int right = arr.size() - 1; // 右边界
while (left <= right) { // 当左指针小于等于右指针时继续循环
int mid = left + (right - left) / 2; // 计算中间索引
if (arr[mid] == target) { // 找到目标,返回索引
return mid;
} else if (arr[mid] < target) { // 如果中间元素小于目标,更新左边界
left = mid + 1;
} else { // 中间元素大于目标,更新右边界
right = mid - 1;
}
}
// 没有找到目标,返回 -1 表示不在数组中
return -1;
}
int main() {
std::vector<int> numbers = {3, 5, 10, 12, 14, 18, 20, 21, 23, 27};
int target = 20;
int result = binarySearch(numbers, target);
if (result != -1) {
std::cout << "Element found at index: " << result << std::endl;
} else {
std::cout << "Element not found in the array." << std::endl;
}
return 0;
}
```
运行此程序,将输出“Element found at index: 6”,因为20在数组中的位置是第6个元素。
阅读全文
相关推荐
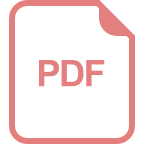
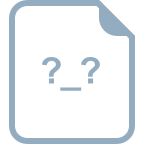
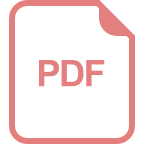

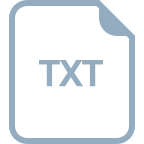
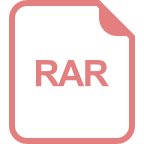
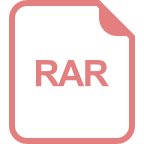
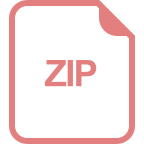
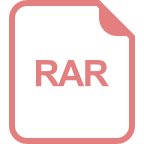
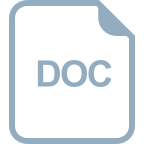
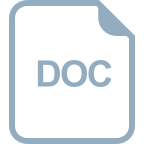
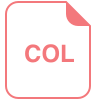



