用两种方式(手动实现、sklearn实现)构建圣保罗市房价数据的线性回归模型,在手动实现的过程中,尝试用图的形式来展示迭代过程中假设函数的变化,设定一个阈值,当参数变化小于阈值时,令梯度下降算法停止。并用jupyter notebook写出相应的代码
时间: 2023-03-27 15:00:28 浏览: 119
好的,我可以回答这个问题。对于圣保罗市房价数据的线性回归模型,我们可以使用手动实现和sklearn实现两种方式。
手动实现的过程中,我们可以使用梯度下降算法来求解模型参数。在迭代过程中,我们可以用图的形式来展示假设函数的变化。设定一个阈值,当参数变化小于阈值时,令梯度下降算法停止。
以下是手动实现的代码示例:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 读取数据
data = pd.read_csv('sao-paulo-properties-april-2019.csv')
# 数据预处理
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
m = len(y)
X = np.hstack((np.ones((m, 1)), X))
theta = np.zeros((X.shape[1], 1))
# 定义代价函数
def compute_cost(X, y, theta):
m = len(y)
J = 1 / (2 * m) * np.sum((X @ theta - y) ** 2)
return J
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, num_iters, threshold):
m = len(y)
J_history = np.zeros((num_iters, 1))
for i in range(num_iters):
h = X @ theta
theta = theta - alpha / m * X.T @ (h - y)
J_history[i] = compute_cost(X, y, theta)
if i > and abs(J_history[i] - J_history[i-1]) < threshold:
break
return theta, J_history[:i+1]
# 运行梯度下降算法
alpha = .01
num_iters = 100
threshold = 1e-5
theta, J_history = gradient_descent(X, y, theta, alpha, num_iters, threshold)
# 绘制代价函数随迭代次数的变化图
plt.plot(range(len(J_history)), J_history)
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.show()
```
对于sklearn实现,我们可以使用LinearRegression模型来求解模型参数。以下是sklearn实现的代码示例:
```python
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression
# 读取数据
data = pd.read_csv('sao-paulo-properties-april-2019.csv')
# 数据预处理
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 训练模型
model = LinearRegression()
model.fit(X, y)
# 输出模型参数
print('Intercept:', model.intercept_)
print('Coefficients:', model.coef_)
```
相关推荐
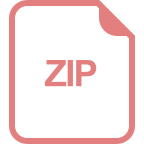
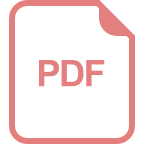








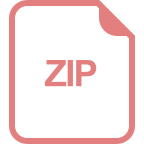
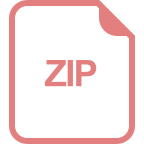
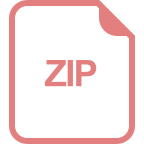
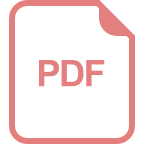
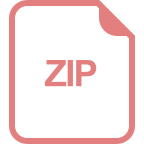