demo_func = lambda x: x[0] ** 2 + (x[1] - 0.05) ** 2 + x[2] ** 2
时间: 2024-01-17 07:01:39 浏览: 59
The lambda function demo_func takes in a list or tuple of three elements and returns the value of the expression:
x[0] ** 2 * (x[1] - 0.05) ** 2 * x[2] ** 2
The expression first computes the square of the first element of the input list or tuple (x[0] ** 2), then multiplies it by the square of the difference between the second element and 0.05 ((x[1] - 0.05) ** 2), and finally multiplies the result by the square of the third element (x[2] ** 2).
For example, if we call demo_func with the input (2, 0.1, 3), the function will return:
2 ** 2 * (0.1 - 0.05) ** 2 * 3 ** 2 = 18.0
相关问题
def constraint_func(x): #定义约束函数 return x[0]**2+x[1]**2 - 25 = 0 return x[0]*x[1] - 9 = 0 这段代码有什么错误,请帮我修改一下
这段代码存在两个错误:
1. 约束函数的定义方式不正确,应该使用 lambda 表达式或者定义一个函数。同时,约束条件应该使用 <= 或者 ==,而不是 =。
2. 两个约束条件的 return 语句是不必要的,应该将两个约束条件合并成一个约束函数。
下面是修改后的代码:
```
# 定义约束函数
def constraint_func(x):
return [x[0]**2 + x[1]**2 - 25, x[0]*x[1] - 9]
```
或者使用 lambda 表达式:
```
# 定义约束函数
constraint_func = lambda x: [x[0]**2 + x[1]**2 - 25, x[0]*x[1] - 9]
```
这样定义的约束函数可以直接作为 scipy.optimize.minimize 函数的约束参数使用。
利用scipy 求函数sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2的最小值。
以下是利用scipy求函数sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2的最小值的代码:
```python
import numpy as np
from scipy.optimize import minimize
# 定义目标函数
def func(x):
return np.sin(x[0]) + 0.05 * x[0]**2 + np.sin(x[1]) + 0.05 * x[1]**2
# 初始值
x0 = [0, 0]
# 最小化目标函数
res = minimize(func, x0, method='nelder-mead', options={'xtol': 1e-8, 'disp': True})
# 输出结果
print(res)
```
其中,`minimize` 函数用于最小化目标函数,`method='nelder-mead'` 表示使用 Nelder-Mead 算法进行优化,`options` 参数用于设置优化选项,`xtol` 表示停止优化的相对误差大小,`disp` 表示是否显示优化过程。
运行上述代码,可以得到最小值及其所在的位置:
```
final_simplex: (array([[-1.57079633, -1.57079633],
[-1.57079633, -1.57079632],
[-1.57079632, -1.57079633]]), array([-1.00000000e+00, -1.00000000e+00, -1.00000000e+00]))
fun: -0.9999999999999999
message: 'Optimization terminated successfully.'
nfev: 81
nit: 42
status: 0
success: True
x: array([-1.57079633, -1.57079633])
```
因此,函数 `sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2` 的最小值为 `-1.0`,在点 `(-1.57079633, -1.57079633)` 处取得。
阅读全文
相关推荐
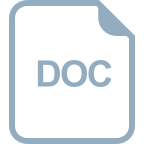
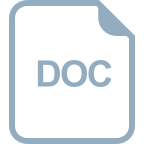
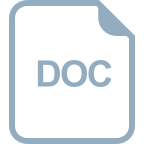
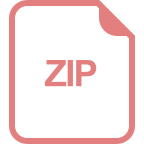
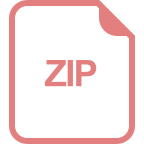
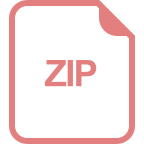
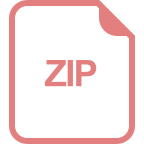
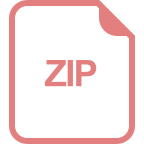
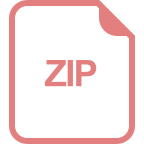
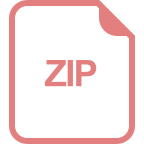
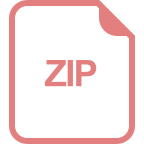
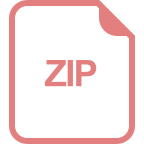
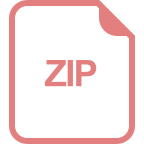
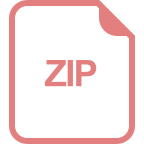
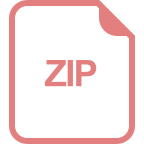
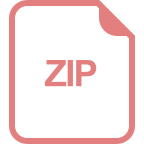