C++如何新建文件夹
时间: 2024-10-18 11:02:55 浏览: 18
在C++中,直接操作操作系统级别的文件和目录功能通常需要使用系统的API或库函数,如Windows API(CreateDirectory)或POSIX标准下的mkdir函数。以下是一个在Windows系统上创建新文件夹的基本示例,使用了C++ Win32 API:
```cpp
#include <windows.h>
#include <iostream>
bool createFolder(const std::wstring& path) {
DWORD result = CreateDirectory(path.c_str(), NULL);
if (result == 0 && GetLastError() != ERROR_ALREADY_EXISTS) {
std::wcout << L"Failed to create directory. Error code: " << GetLastError() << std::endl;
return false;
}
return true;
}
int main() {
std::wstring folderPath(L"C:\\NewFolder");
if (!createFolder(folderPath)) {
std::wcout << L"Failed to create the folder." << std::endl;
} else {
std::wcout << L"Successfully created the folder." << std::endl;
}
return 0;
}
```
对于Linux或Unix-like系统,可以使用`boost/filesystem`库或者C++17引入的`std::filesystem`库:
```cpp
#include <boost/filesystem.hpp> // 或 #include <filesystem>
using namespace boost::filesystem; // 或 std
void createFolder(const std::string& path) {
try {
create_directory(path);
std::cout << "Successfully created the folder: " << path << std::endl;
} catch (const filesystem_error &e) {
std::cerr << "Failed to create the folder. Reason: " << e.what() << std::endl;
}
}
int main() {
std::string folderPath = "/home/user/NewFolder";
createFolder(folderPath);
return 0;
}
```
阅读全文
相关推荐
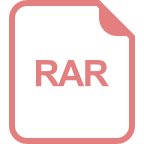
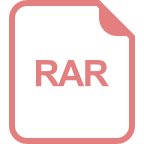



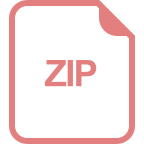
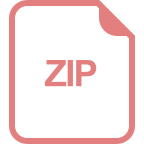
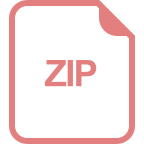
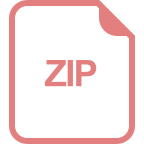
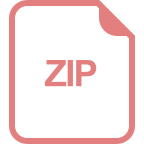
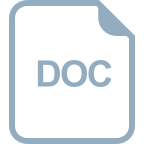




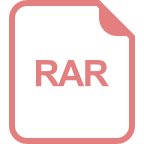